laravel 读取文件
时间: 2023-06-21 10:13:24 浏览: 50
在 Laravel 中,可以使用 `Storage` 门面来读取文件。首先,确保你已经在 `config/filesystems.php` 文件中定义了使用的磁盘。默认情况下,Laravel 使用 `local` 磁盘。
接着,你可以使用 `Storage::get($path)` 方法来读取文件的内容,其中 `$path` 参数是文件的路径。例如:
```php
use Illuminate\Support\Facades\Storage;
$content = Storage::get('file.txt');
```
如果你想逐行读取文件内容,可以使用 `Storage::readLines($path)` 方法。例如:
```php
use Illuminate\Support\Facades\Storage;
$lines = Storage::readLines('file.txt');
foreach ($lines as $line) {
echo $line;
}
```
另外,如果你需要读取远程文件,可以使用 `Storage::disk('s3')->get('file.jpg')` 方法,并指定使用的磁盘。例如:
```php
use Illuminate\Support\Facades\Storage;
$content = Storage::disk('s3')->get('file.jpg');
```
相关问题
laravel 读取excel表内容
在 Laravel 中,你可以使用 `Maatwebsite\Excel` 扩展包来读取 Excel 表格的内容。下面是一个简单的示例代码,演示了如何在 Laravel 中读取 Excel 表格内容:
1. 首先,安装 `Maatwebsite\Excel` 扩展包:
```bash
composer require maatwebsite/excel
```
2. 创建一个控制器,并在其中编写读取 Excel 表格的逻辑。假设你已经创建了一个名为 `ImportController` 的控制器,可以在其中添加以下代码:
```php
<?php
namespace App\Http\Controllers;
use Maatwebsite\Excel\Facades\Excel;
class ImportController extends Controller
{
public function import()
{
$path = public_path('path_to_your_excel_file.xlsx');
$data = Excel::import($path)->get();
// 处理读取到的数据
foreach ($data as $row) {
// 操作每一行数据
// $row 是一个关联数组,包含了每一行的字段和值
// 例如 $row['name'], $row['email']
}
// 返回结果或进行其他操作
}
}
```
3. 在路由文件 `web.php` 中添加一个路由,将请求映射到 `ImportController` 的 `import()` 方法:
```php
Route::get('/import', 'ImportController@import');
```
4. 最后,通过访问 `/import` 路由来触发读取 Excel 表格的操作。
请注意,上述示例中的 `path_to_your_excel_file.xlsx` 应该替换为你实际存放 Excel 文件的路径。另外,你可能需要根据 Excel 表格的具体结构来调整代码以适应你的需求。
Laravel excel
Laravel Excel是一个在Laravel 5中使用的库,它提供了导入和导出Excel/CSV文件的功能。通过使用Laravel Excel,你可以轻松地读取和写入Excel文件,并且可以方便地处理数据。
要在Laravel 5中使用Laravel Excel,你可以按照以下步骤进行操作:
1. 首先,你需要使用Composer来安装Laravel Excel。可以使用以下命令在你的Laravel项目中安装Laravel Excel:
```
composer require maatwebsite/excel
```
2. 安装完成后,在config/app.php文件中的providers数组中添加以下内容:
```
Maatwebsite\Excel\ExcelServiceProvider::class,
```
还需要在aliases数组中添加以下内容:
```
'Excel' => Maatwebsite\Excel\Facades\Excel::class,
```
3. 接下来,你可以创建一个控制器来处理Excel文件的导入和导出逻辑。在控制器中,你可以使用Laravel Excel提供的一些方法来读取和写入Excel文件。以下是一些示例代码:
```php
use Excel;
// 导出Excel文件
public function export()
{
$data = [
['Name', 'Email'],
['John Doe', 'john@example.com'],
['Jane Smith', 'jane@example.com'],
];
return Excel::download(function($excel) use ($data) {
$excel->setTitle('Users');
$excel->sheet('Sheet 1', function($sheet) use ($data) {
$sheet->fromArray($data, null, 'A1', false, false);
});
}, 'users.xlsx');
}
// 导入Excel文件
public function import(Request $request)
{
$file = $request->file('file');
Excel::import(function($excel) {
$excel->sheet(0, function($sheet) {
$data = $sheet->toArray();
// 处理导入的数据
foreach($data as $row) {
// ...
}
});
}, $file);
}
```
通过这些代码示例,你可以在Laravel中使用Laravel Excel来实现Excel/CSV文件的导入和导出功能。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [Laravel 5使用Laravel Excel实现Excel/CSV文件导入导出的功能详解](https://download.csdn.net/download/weixin_38617196/12962745)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
- *2* *3* [laravel-excel使用(老猫包子店的故事)](https://blog.csdn.net/qq_39071185/article/details/122015370)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐
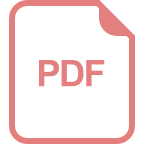












