PHPEXcel和easyui采用MVC框架写一个超大Excel上传到数据库,先插入临时表,根据临时的字段查入实际的数据库,用临时的某个字段是字段判断以前的某个字段重复跳过下一行插入数据并且在上传完显示到上界面
时间: 2024-04-16 17:24:49 浏览: 140
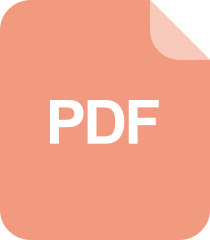
利用phpexcel把excel导入数据库和数据库导出excel实现
您可以按照以下步骤来实现您的需求:
1. 首先,创建一个MVC架构的项目。您可以使用任何流行的PHP框架,如Laravel、CodeIgniter或Yii等。
2. 在您的视图层中,使用easyui创建一个上传文件的表单,并将表单数据发送到控制器。
3. 在控制器中,接收上传文件,并使用PHPEXcel库读取Excel文件数据。您可以使用PHPEXcel的方法,如`load()`函数来加载Excel文件。
4. 在控制器中,创建一个临时表来存储Excel数据。您可以使用数据库操作类或原生SQL语句来执行插入操作,并将Excel数据插入到临时表中。
5. 接下来,根据临时表中的字段进行查询操作。您可以使用SQL语句来查询具有重复字段的数据行。
6. 根据查询结果,在实际数据库中插入数据。您可以使用数据库操作类或原生SQL语句来执行插入操作,并将数据插入到实际的数据库表中。
7. 最后,将上传数据显示在前端界面上。您可以在控制器中查询实际数据库,并将结果传递给视图层,然后使用easyui来渲染和显示数据。
请注意,以上步骤是一个大致的指导,具体实现还需要根据您所选择的框架和库进行调整。此外,代码的安全性和数据验证也是非常重要的,您可能需要添加适当的验证和过滤来确保数据的准确性和安全性。
阅读全文
相关推荐
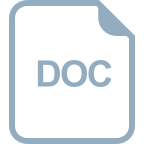
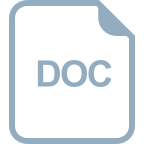




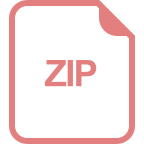
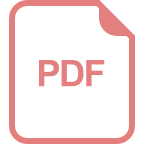
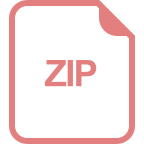
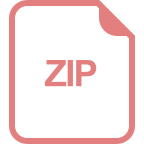
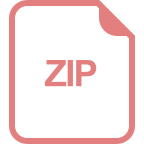
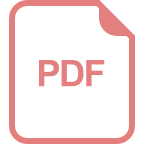
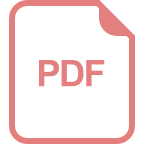
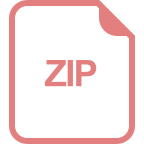
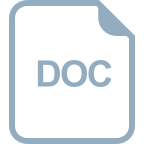

