写一个excel上传到数据库展示到前端,要求采用MVC,前端框架easyui
时间: 2024-04-17 22:26:11 浏览: 123
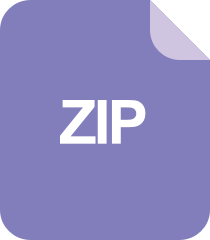
使用MVC+easyui

Sure! Here's an example of how you can upload an Excel file, store its data in a database, and display it on the front-end using the MVC pattern and the EasyUI framework:
1. Create the necessary database table to store the Excel data. Let's assume the table is named `excel_data` and has columns `id`, `name`, and `email`.
2. Create a file named `index.php` for the front-end view using EasyUI:
```html
<!DOCTYPE html>
<html>
<head>
<title>Excel Upload</title>
<link rel="stylesheet" type="text/css" href="https://cdn.jsdelivr.net/npm/easyui@1.9.25/themes/default/easyui.css">
<script type="text/javascript" src="https://cdn.jsdelivr.net/npm/jquery/dist/jquery.min.js"></script>
<script type="text/javascript" src="https://cdn.jsdelivr.net/npm/easyui@1.9.25/jquery.easyui.min.js"></script>
</head>
<body>
<h2>Excel Upload</h2>
<form id="excelForm" method="post" enctype="multipart/form-data">
<input id="excelFile" name="excelFile" type="file">
<button id="uploadBtn" class="easyui-linkbutton" type="submit">Upload</button>
</form>
<table id="datagrid"></table>
<script type="text/javascript">
$(function(){
$('#excelForm').form({
url: 'upload.php',
onSubmit: function(){
return $(this).form('validate');
},
success: function(result){
$('#datagrid').datagrid('reload');
$.messager.show({
title: 'Success',
msg: result,
timeout: 5000
});
}
});
$('#datagrid').datagrid({
url: 'data.php',
fitColumns: true,
columns: [[
{field: 'id', title: 'ID', width: 50},
{field: 'name', title: 'Name', width: 100},
{field: 'email', title: 'Email', width: 150}
]]
});
});
</script>
</body>
</html>
```
3. Create a file named `upload.php` to handle the file upload and data insertion into the database:
```php
<?php
require_once 'PHPExcel/PHPExcel.php';
// Database connection details
$host = 'localhost';
$username = 'your_username';
$password = 'your_password';
$database = 'your_database';
// File upload directory
$targetDir = 'uploads/';
if (!is_dir($targetDir)) {
mkdir($targetDir, 0777, true);
}
$targetFile = $targetDir . basename($_FILES["excelFile"]["name"]);
move_uploaded_file($_FILES["excelFile"]["tmp_name"], $targetFile);
$objPHPExcel = PHPExcel_IOFactory::load($targetFile);
$worksheet = $objPHPExcel->getActiveSheet();
$conn = new mysqli($host, $username, $password, $database);
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
foreach ($worksheet->getRowIterator() as $row) {
$rowData = [];
$cellIterator = $row->getCellIterator();
$cellIterator->setIterateOnlyExistingCells(false);
foreach ($cellIterator as $cell) {
$rowData[] = $cell->getValue();
}
$sql = "INSERT INTO excel_data (name, email) VALUES (?, ?)";
$stmt = $conn->prepare($sql);
$stmt->bind_param("ss", $rowData[0], $rowData[1]);
$stmt->execute();
}
$conn->close();
unlink($targetFile);
echo "Upload successful!";
?>
```
4. Create a file named `data.php` to fetch the data from the database and return it as JSON for the front-end grid:
```php
<?php
// Database connection details
$host = 'localhost';
$username = 'your_username';
$password = 'your_password';
$database = 'your_database';
$conn = new mysqli($host, $username, $password, $database);
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
$sql = "SELECT * FROM excel_data";
$result = $conn->query($sql);
$data = array();
while ($row = $result->fetch_assoc()) {
$data[] = $row;
}
$conn->close();
echo json_encode($data);
?>
```
Make sure to replace `'your_username'`, `'your_password'`, and `'your_database'` with your actual database credentials.
This example follows the MVC pattern by separating the front-end view (`index.php`), the controller (`upload.php`), and the model (`data.php`).
Remember to include the PHPExcel library in the appropriate locations and adjust the file paths accordingly.
I hope this example helps you with uploading an Excel file, storing the data in a database, and displaying it on the front-end using the MVC pattern and EasyUI framework. Let me know if you have any further questions!
阅读全文
相关推荐
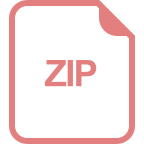

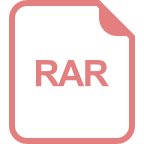
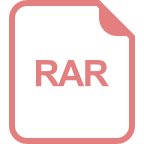
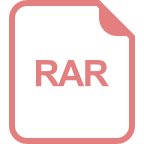
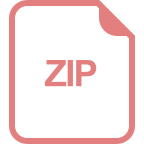
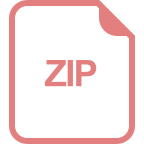
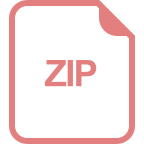
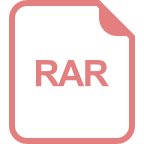
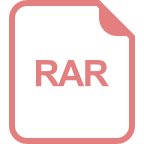
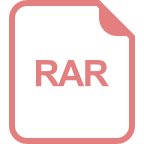
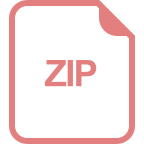
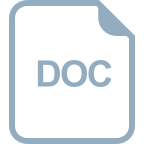
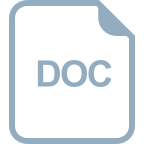
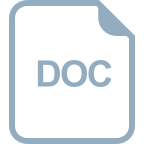
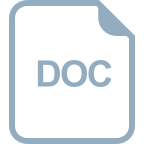
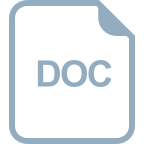