写一个PHPexcel上传到MySQL数据库,要求采用前后端分离,前端框架easyui
时间: 2024-04-17 19:26:06 浏览: 132
Sure, I can help you with that. Here's an example of how you can upload a PHPExcel file to a MySQL database using a front-end framework like EasyUI in a separate front-end and back-end setup.
First, let's start with the front-end code using EasyUI to handle the file upload:
```html
<!DOCTYPE html>
<html>
<head>
<title>Excel Upload</title>
<link rel="stylesheet" type="text/css" href="https://cdn.jsdelivr.net/npm/easyui@1.9.25/themes/default/easyui.css">
<script type="text/javascript" src="https://cdn.jsdelivr.net/npm/jquery/dist/jquery.min.js"></script>
<script type="text/javascript" src="https://cdn.jsdelivr.net/npm/easyui@1.9.25/jquery.easyui.min.js"></script>
</head>
<body>
<h2>Excel Upload</h2>
<form id="excelForm" method="post" enctype="multipart/form-data">
<input id="excelFile" name="excelFile" type="file">
<button id="uploadBtn" class="easyui-linkbutton" type="submit">Upload</button>
</form>
<script type="text/javascript">
$(function(){
$('#excelForm').form({
url: 'upload.php',
onSubmit: function(){
return $(this).form('validate');
},
success: function(result){
$.messager.show({
title: 'Success',
msg: result,
timeout: 5000
});
}
});
});
</script>
</body>
</html>
```
Next, let's create the `upload.php` file on the server side to handle the file upload and database insertion:
```php
<?php
// Include PHPExcel library
require_once 'PHPExcel/PHPExcel.php';
// Database connection details
$host = 'localhost';
$username = 'your_username';
$password = 'your_password';
$database = 'your_database';
// File upload directory
$targetDir = 'uploads/';
// Create target directory if it doesn't exist
if (!is_dir($targetDir)) {
mkdir($targetDir, 0777, true);
}
// Handle file upload
$targetFile = $targetDir . basename($_FILES["excelFile"]["name"]);
move_uploaded_file($_FILES["excelFile"]["tmp_name"], $targetFile);
// Read the uploaded file using PHPExcel
$objPHPExcel = PHPExcel_IOFactory::load($targetFile);
$worksheet = $objPHPExcel->getActiveSheet();
// Database connection
$conn = new mysqli($host, $username, $password, $database);
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// Loop through each row in the worksheet
foreach ($worksheet->getRowIterator() as $row) {
$rowData = [];
$cellIterator = $row->getCellIterator();
$cellIterator->setIterateOnlyExistingCells(false);
// Loop through each cell in the row
foreach ($cellIterator as $cell) {
$rowData[] = $cell->getValue();
}
// Insert row data into the database
$sql = "INSERT INTO your_table_name (column1, column2, column3) VALUES (?, ?, ?)";
$stmt = $conn->prepare($sql);
$stmt->bind_param("sss", $rowData[0], $rowData[1], $rowData[2]);
$stmt->execute();
}
// Close database connection
$conn->close();
// Delete the uploaded file
unlink($targetFile);
echo "Upload successful!";
?>
```
Make sure to create a directory named "uploads" in the same directory as the `upload.php` file to store the uploaded files.
Replace `'your_username'`, `'your_password'`, `'your_database'`, and `'your_table_name'` with your actual database credentials and table name.
This is a basic example to get you started. You may need to modify it based on your specific requirements and validations. Additionally, make sure to sanitize and validate user input to prevent any security vulnerabilities.
I hope this helps you with uploading PHPExcel files to a MySQL database using a front-end framework like EasyUI in a separate front-end and back-end setup. Let me know if you have any further questions!
阅读全文
相关推荐
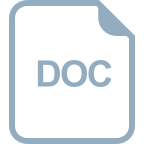
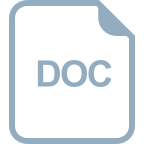
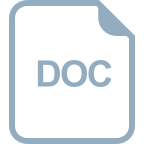

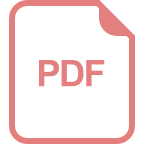



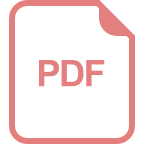
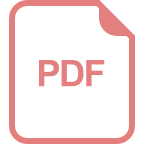
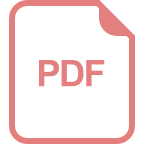
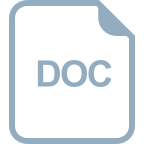




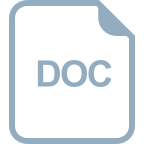
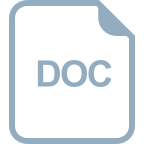
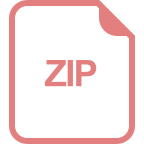
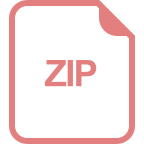