如何在 python 子区域中绘制一个多条折线的折线图
时间: 2024-03-23 20:43:20 浏览: 32
您可以使用Matplotlib库来在python子区域中绘制多条折线的折线图。具体步骤如下:
1. 导入Matplotlib库
```python
import matplotlib.pyplot as plt
```
2. 创建一个Figure对象和一个Axes对象
```python
fig, ax = plt.subplots()
```
3. 在Axes对象中绘制多条折线图
```python
ax.plot(x1, y1)
ax.plot(x2, y2)
...
```
其中,x1、x2、...、xn和y1、y2、...、yn分别表示多条折线的横纵坐标数据。
4. 设置子区域的坐标范围
```python
ax.set_xlim(xmin, xmax)
ax.set_ylim(ymin, ymax)
```
其中,xmin、xmax、ymin、ymax分别表示子区域的横纵坐标范围。
完整代码示例:
```python
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y1 = [2, 4, 6, 8, 10]
y2 = [1, 3, 5, 7, 9]
fig, ax = plt.subplots()
ax.plot(x, y1)
ax.plot(x, y2)
ax.set_xlim(1, 5)
ax.set_ylim(1, 10)
plt.show()
```
运行代码后,会在屏幕上显示一个子区域绘制的多条折线的折线图。
相关问题
如何在Python绘图区域的子区域绘制一个条折线的折线图
您可以使用Matplotlib库来完成这个任务。具体步骤如下:
1. 导入Matplotlib库
```python
import matplotlib.pyplot as plt
```
2. 创建一个Figure对象和一个Axes对象
```python
fig, ax = plt.subplots()
```
3. 在Axes对象中绘制折线图
```python
ax.plot(x, y)
```
其中,x和y表示折线的横纵坐标数据。
4. 设置子区域的坐标范围
```python
ax.set_xlim(xmin, xmax)
ax.set_ylim(ymin, ymax)
```
其中,xmin、xmax、ymin、ymax分别表示子区域的横纵坐标范围。
完整代码示例:
```python
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
fig, ax = plt.subplots()
ax.plot(x, y)
ax.set_xlim(1, 3)
ax.set_ylim(2, 6)
plt.show()
```
运行代码后,会在屏幕上显示一个子区域绘制的折线图。
python一个图中绘制多个折线图
可以使用matplotlib库来实现在一个图中绘制多个折线图。
首先,导入必要的库和生成数据:
```python
import matplotlib.pyplot as plt
import numpy as np
# 生成数据
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
y3 = np.tan(x)
```
然后,创建一个figure对象和axes对象:
```python
fig, ax = plt.subplots()
```
接着,在ax对象上绘制三个折线图:
```python
ax.plot(x, y1, label='sin(x)')
ax.plot(x, y2, label='cos(x)')
ax.plot(x, y3, label='tan(x)')
```
最后,添加图例和设置标题等:
```python
ax.legend()
ax.set_title('Multiple Line Plots')
ax.set_xlabel('x')
ax.set_ylabel('y')
plt.show()
```
完整代码如下:
```python
import matplotlib.pyplot as plt
import numpy as np
# 生成数据
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
y3 = np.tan(x)
# 创建figure对象和axes对象
fig, ax = plt.subplots()
# 在ax对象上绘制三个折线图
ax.plot(x, y1, label='sin(x)')
ax.plot(x, y2, label='cos(x)')
ax.plot(x, y3, label='tan(x)')
# 添加图例和设置标题等
ax.legend()
ax.set_title('Multiple Line Plots')
ax.set_xlabel('x')
ax.set_ylabel('y')
# 显示图像
plt.show()
```
相关推荐
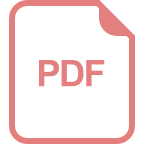
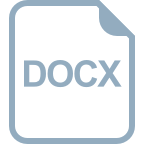
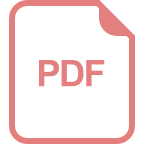
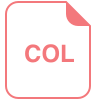
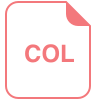
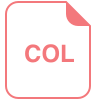
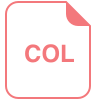
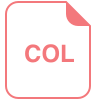







