app.post('/admin/reviewClaim', async (req, res) => { try { const { _id, state } = req.body; await Lose.findByIdAndUpdate(_id, { state }); res.send("success"); } catch (error){ console.log(error); res.send("error"); } })根据用户认领情况生成Vue数据统计折线图功能代码
时间: 2024-02-11 16:09:27 浏览: 30
生成根据用户认领情况生成Vue数据统计折线图的功能代码需要以下步骤:
1. 安装 Vue 和 Chart.js 库。
```bash
npm install vue chart.js --save
```
2. 在 Vue 组件中引入 Chart.js 库和样式文件。
```javascript
import Chart from 'chart.js';
import 'chart.js/dist/chart.min.css'
```
3. 在 Vue 组件的模板中添加一个 canvas 元素,用于渲染折线图。
```html
<template>
<div>
<canvas ref="chart"></canvas>
</div>
</template>
```
4. 在 Vue 组件的脚本中定义一个方法,用于获取用户认领数据,并将数据转换为 Chart.js 所需的格式。
```javascript
methods: {
async getUserClaimStats() {
try {
const res = await axios.get('/api/userClaims');
const data = res.data;
const labels = data.map(item => item.date);
const counts = data.map(item => item.count);
return { labels, counts };
} catch (error) {
console.log(error);
return { labels: [], counts: [] };
}
}
}
```
5. 在 Vue 组件的脚本中定义一个钩子函数,在组件加载时获取用户认领数据,并使用 Chart.js 绘制折线图。
```javascript
mounted() {
this.getUserClaimStats().then(({ labels, counts }) => {
const ctx = this.$refs.chart.getContext('2d');
const chart = new Chart(ctx, {
type: 'line',
data: {
labels: labels,
datasets: [{
label: '认领次数',
data: counts,
fill: false,
borderColor: 'rgb(75, 192, 192)',
tension: 0.1
}]
},
options: {
responsive: true,
maintainAspectRatio: false
}
});
});
}
```
在这个示例代码中,我们使用了 axios 库发送了一个 GET 请求到指定的 URL,获取用户认领数据,并在组件加载时将数据转换为 Chart.js 所需的格式,并使用 Chart.js 绘制了一个折线图。
相关推荐
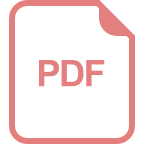
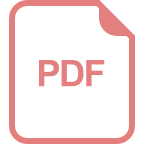
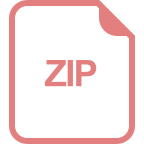











const fs = require("fs"); const http = require("http"); const {promisify} = require("util"); const path = require("path"); let server = http.createServer(async (req,res)=>{ let urlObj = new URL(req.url,"http://"+req.headers.host); let removeDir = require("./removedir") let {pathname}=urlObj; res.setHeader("Content-Type","text/html;charset=utf-8") if(pathname ==="/"){ let pathurl = "./";/* 当前目录 */ let showurl = urlObj.searchParams.get("showurl"); if(showurl){ pathurl = showurl; } /* 判断是否为文件 */ let stat = fs.statSync(pathurl); if (stat.isFile()) { // 是文件 let filedata = await promisify(fs.readFile)(pathurl); res.write(filedata); res.end(); } // 判断是否需要执行删除的逻辑 let delpath = urlObj.searchParams.get("delpath"); if (delpath) { // 有 delpath参数 ,那么证明需要删除逻辑; if (fs.existsSync(delpath)) { let stat = fs.statSync(delpath); if (stat.isFile()) { // 是文件 : fs.unlinkSync(delpath); } else { // 是目录 removeDir(delpath); } } } let resdata = await promisify(fs.readdir)(pathurl); let tablestr = "文件名 创建时间 操作 "; resdata.forEach(item=>{ let myurl = path.join(pathurl,item); let stat = fs.statSync(myurl); console.log(stat); tablestr += ${item} ${stat.birthtime} 删除 }) //console.log(tablestr,"????????????????????????????") tablestr += ""; res.write(tablestr); res.end(); } } ) server.listen(8888);




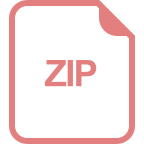