app.post('/toClaim', async (req, res) => { try { const { desc, img_url, openid, _id } = req.body; await Lose.findByIdAndUpdate(_id, { claimInfo: { desc, img_url, openid }, state: 1 }); res.send("success"); } catch (error) { console.log(error); res.send("error"); } })
时间: 2023-06-26 10:04:48 浏览: 45
这段代码使用 Express 框架中的 app.post 方法,监听客户端发送过来的 POST 请求,请求地址为 /toClaim。在请求处理函数中,首先使用解构赋值获取客户端发送过来的请求体中的 desc、img_url、openid、_id 四个属性。然后使用 Mongoose 提供的静态方法 findByIdAndUpdate,根据 _id 更新数据库中的一条数据,将其 claimInfo 字段设置为一个对象,该对象包含 desc、img_url、openid 三个属性,将 state 字段设置为 1。最后向客户端发送字符串 "success" 或 "error",表示请求处理结果。如果在执行过程中发生错误,会将错误信息打印到控制台,并向客户端发送 "error" 字符串。
相关问题
app.post('/toCliam', async(req, res) => { try { const { desc, img_url, openid, _id} = req.body await Lose.findByIdAndUpdate(_id, { claimInfo: { desc, img_url, openid }, state: 1 }); res.send("success"); } catch(error){ console.log(error); res.send("error"); } })如果state为1则生成6位数取件码
是的,您可以在更新状态为1的时候生成6位数取件码。可以参考如下代码:
```javascript
app.post('/toClaim', async(req, res) => {
try {
const { desc, img_url, openid, _id } = req.body;
const code = Math.floor(Math.random() * 900000 + 100000);
await Lose.findByIdAndUpdate(_id, {
claimInfo: {
desc,
img_url,
openid,
code // 将生成的6位数取件码存储到数据库中
},
state: 1
});
res.send("success");
} catch(error) {
console.log(error);
res.send("error");
}
})
```
在这个代码中,我们使用了`Math.random()`方法来生成一个6位数的随机数,然后将其存储到数据库中。这个方法生成的随机数不一定是6位数,因此我们需要使用`Math.floor()`方法向下取整,再加上一个100000,这样就可以保证生成的随机数一定是6位数了。
app.post('/toClaim', async(req, res) => { try { const { desc, img_url, openid, _id } = req.body; const code = Math.floor(Math.random() * 900000 + 100000); await Lose.findByIdAndUpdate(_id, { claimInfo: { desc, img_url, openid, code , }, state: 1 }); res.send("success"); } catch(error) { console.log(error); res.send("error"); } }) toClaim() { this.setData({ showModal: true }) },如何认领中获取后端的code
在前端调用 `toClaim()` 函数时,可以在函数内使用 `wx.request()` 方法向后端发送请求,在请求的回调函数中,将后端返回的数据中的 `code` 属性赋值给前端的某个变量,以供后续使用。示例代码如下:
```
toClaim() {
wx.request({
url: '后端接口地址',
method: 'POST',
data: {
// 请求参数
},
success: (res) => {
// 请求成功回调函数
if (res.data === 'success') {
// 获取 code 属性并保存到前端的变量中
const code = res.data.claimInfo.code;
this.setData({
showModal: true,
code: code
});
} else {
wx.showToast({
title: '认领失败',
icon: 'none'
});
}
},
fail: (err) => {
// 请求失败回调函数
console.log(err);
wx.showToast({
title: '请求失败',
icon: 'none'
});
}
});
}
```
相关推荐
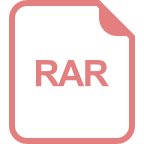





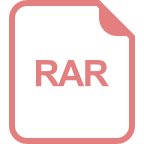
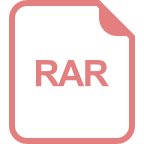
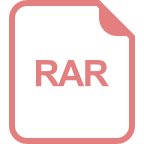
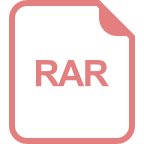
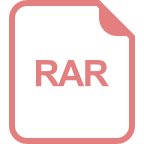