编写一个Linux C程序,它接受命令行输入参数并使用getopt函数解析选项。
时间: 2024-09-30 17:03:33 浏览: 29
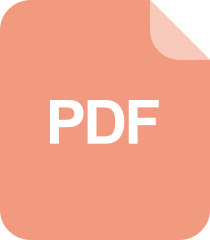
20120906_命令行参数解析1
编写一个Linux C程序来处理命令行输入参数并使用`getopt`函数解析选项,通常涉及到以下几个步骤:
1. 包含头文件:首先,你需要包含`stdio.h`、`stdlib.h`和`unistd.h`,以及`getopt.h`,后者提供了`getopt`函数。
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <getopt.h>
```
2. 定义结构体和标志字典:创建一个结构体来存储用户指定的选项及其值,然后定义一个`char *opt_string[]`数组,其中元素对应于`getopt_long()`函数使用的选项标记。
```c
struct options {
int arg1;
char *arg2;
// 其他可能的选项...
};
#define OPTION_LIST "a:b:h" // 这里'a', 'b' 和 'h'是示例选项
static struct options opt;
```
3. 主函数和`main()`:在`main()`函数中,设置程序名称作为参数给`getopt()`,并初始化标志字典。
```c
int main(int argc, char *argv[]) {
const char* progname = argv[0];
int opt_char;
while ((opt_char = getopt_long(argc, argv, OPTION_LIST, NULL, NULL)) != -1) {
switch (opt_char) {
case 'a':
opt.arg1 = atoi(optarg);
break;
case 'b':
opt.arg2 = optarg;
break;
case 'h': // 帮助选项
usage();
return 0;
default:
fprintf(stderr, "Unknown option %c\n", opt_char);
usage();
return 1;
}
}
// 接下来的代码处理剩余参数...
}
```
4. 辅助函数:创建一个名为`usage()`的函数,用于显示帮助信息和程序如何使用选项。
```c
void usage() {
printf("Usage: %s [options]\n", progname);
printf("Options:\n");
printf("\t-a <number>\n");
printf("\t-b <string>\n");
printf("\t-h\tShow this help message.\n");
}
```
5. 结束主循环并处理参数:在`getopt_long()`返回 `-1` 或最后一个选项处理完之后,可以开始处理剩余的非选项参数。
现在,当你运行这个程序并提供相应的命令行选项时,`getopt()`会解析它们并将结果存储在`opt`结构体中。
阅读全文
相关推荐
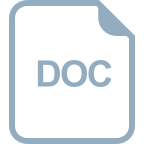
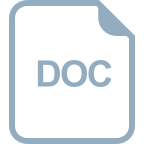

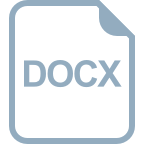
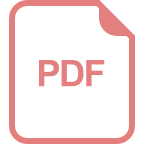
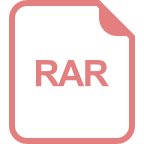
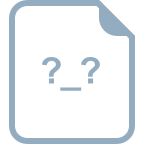
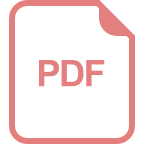
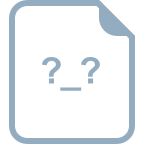
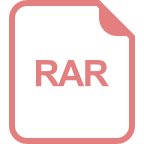
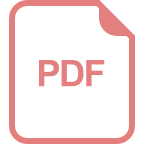
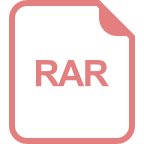
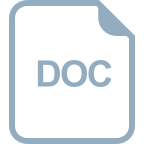
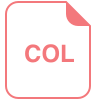
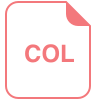
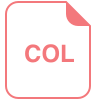

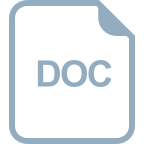
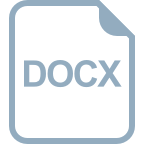