请告诉我如何用Pycharm对一段句子进行预处理?
时间: 2024-10-10 12:03:14 浏览: 20
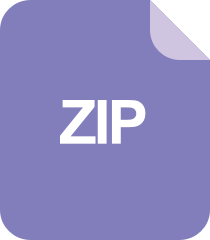
pycharm安装教程tention-model-for-n开发笔记
在PyCharm中进行文本预处理,一般包括以下几个步骤:
1. **导入所需库**:首先需要安装一些用于文本处理的库,比如`nltk`(自然语言工具包)、`spaCy`或`Pandas`。你可以通过PyCharm的集成终端或环境管理器安装它们。
2. **加载数据**:将需要预处理的文本读入到程序中,可以是一个字符串变量、文件内容或整个CSV/JSON文件中的数据。
```python
import pandas as pd
# 从文件读取
with open('your_file.txt', 'r') as file:
text = file.read()
```
3. **分词(Tokenization)**:将句子分解成单词或短语。
```python
from nltk.tokenize import word_tokenize
words = word_tokenize(text)
```
4. **移除停用词(Removing Stop Words)**:
```python
from nltk.corpus import stopwords
stop_words = set(stopwords.words('your_language'))
filtered_words = [word for word in words if word not in stop_words]
```
5. **转换为小写(Lowercasing)**:统一所有字母为小写,减少拼写形式带来的差异。
```python
words = [word.lower() for word in filtered_words]
```
6. **标点符号和数字移除(Punctuation and Numbers Removal)**:
```python
words = [word.strip(".,!?:;'-") for word in words]
```
7. **其他预处理选项**:可能还包括词干提取(Stemming)、词形还原(Lemmatization)、词性标注(Part-of-Speech Tagging)、标准化特殊字符等操作,这取决于具体任务的需求。
完成以上预处理后,你可以得到一个清洗后的词汇列表,可以用于后续的文本分析或建模。
阅读全文
相关推荐
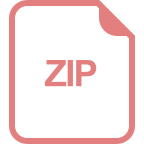
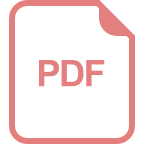


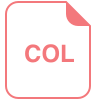












