Python定义2个变量,分别用format(),f-string两种方法输出
时间: 2024-10-19 09:09:35 浏览: 26
在Python中,你可以使用`format()`函数和f-string(格式化字符串字面量)来定义并输出变量。
**使用`format()`函数:**
```python
name = "Alice"
age = 25
# 使用 format() 函数
formatted_string = "My name is {} and I am {} years old.".format(name, age)
print(formatted_string) # 输出:My name is Alice and I am 25 years old.
```
**使用 f-string:**
```python
name = "Bob"
age = 30
# 使用 f-string
formatted_string_fstring = f"My name is {name} and I am {age} years old."
print(formatted_string_fstring) # 输出:My name is Bob and I am 30 years old.
```
这两种方法都可以将变量值插入到字符串中,使输出更具动态性。f-string通常更简洁易读,并且支持更多的表达式和格式选项。不过,`format()`函数在早期版本的Python中更为常见。
相关问题
1.分别使用%,format以及f-string三种方式打印学生信息如下图(输出自己或者同学的个人信息):
当然可以。以下是使用Python的`%`, `format()`, 和 f-string 三种方式来打印学生信息的例子:
1. 使用 `%` 模板:
```python
name = "张三"
age = 18
grade = "一年级"
print("姓名:%s, 年龄:%d, 级别:%s" % (name, age, grade))
```
2. 使用 `format()` 函数:
```python
name = "李四"
age = 20
grade = "二年级"
print("姓名:{},年龄:{},级别:{}".format(name, age, grade))
```
3. 使用 f-string (Python 3.6 及以上版本):
```python
student = {"name": "王五", "age": 21, "grade": "三年级"}
print(f"姓名:{student['name']},年龄:{student['age']},级别:{student['grade']}")
```
这三种方法都可以用来格式化字符串并插入变量值,只是语法有所不同。
f-string的格式化输出方式
f-string是Python3.6引入的一种新的字符串格式化方式,它的核心思想和format一样,但使用起来更加直观和简单。在f-string中,我们可以在字符串前加上字母"f",然后在字符串中使用大括号{}来引用变量或表达式,这些变量或表达式会在运行时被替换为相应的值。例如,可以使用f-string来输出一个变量x的值,代码如下:
```
x = 10
print(f"The value of x is {x}")
```
输出结果为:
```
The value of x is 10
```
除了变量,我们还可以在大括号中使用表达式,例如:
```
a = 10
b = 20
print(f"The sum of {a} and {b} is {a+b}")
```
输出结果为:
```
The sum of 10 and 20 is 30
```
在f-string中,我们还可以使用各种格式化选项来控制输出的格式,例如:
```
x = 3.1415926
print(f"The value of x is {x:.2f}")
```
输出结果为:
```
The value of x is 3.14
```
这里的":.2f"表示输出x的值时保留两位小数。
阅读全文
相关推荐
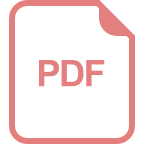
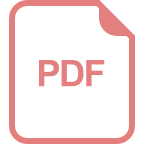
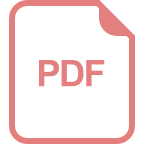
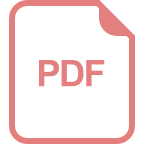
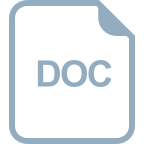
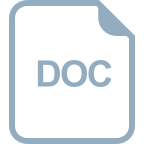
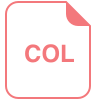
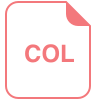
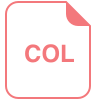
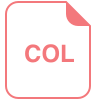
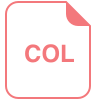
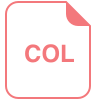
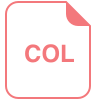
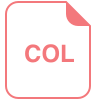

