(1) 建立一个长度为n的单链表。 (2) 插入新结点。 (3) 删除某一个结点。 (4) 打印输出La中的结点元素值。
时间: 2024-10-21 15:11:01 浏览: 34
建立一个长度为n的单链表通常涉及以下几个步骤:
1. **创建头节点**:首先,需要创建一个名为`Node`的结构体或类,它包含两个属性,一个是数据域用于存储元素值,另一个是指向下一个节点的指针。然后创建一个空链表,即头节点`head`设置为`None`。
```python
class Node:
def __init__(self, value=None):
self.value = value
self.next = None
# 初始化空链表
head = None
```
2. **插入新节点**:对于任意位置的插入,一般会找到插入点并更新其指向。例如,如果要在末尾插入,可以创建新节点并将其next指向当前尾节点,然后将尾节点的next更新为新节点。
```python
def insert_at_end(head, value):
new_node = Node(value)
if not head:
head = new_node
else:
current = head
while current.next:
current = current.next
current.next = new_node
return head
```
3. **删除某个节点**:若要删除特定值的节点,你需要遍历链表找到该节点,并更新前一个节点的next指针跳过它。注意,如果没有找到要删除的节点,需要处理异常或返回错误信息。
```python
def delete_value(head, value):
if not head:
return head
if head.value == value:
return head.next
prev = head
curr = head.next
while curr and curr.value != value:
prev = curr
curr = curr.next
if curr is None:
return head
prev.next = curr.next
return head
```
4. **打印链表元素**:遍历整个链表,逐个访问每个节点的值并输出。
```python
def print_list(head):
current = head
while current:
print(current.value, end=" -> ")
current = current.next
print("None") # 结束标记
```
阅读全文
相关推荐
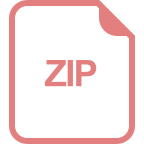
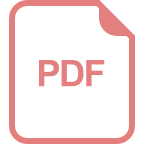
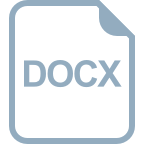










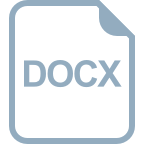
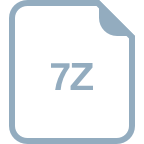