(1) 建立一个长度为n的单链表。 (2) 插入新结点。 (3) 删除某一个结点。 (4) 打印输出La中的结点元素值。
时间: 2024-10-21 14:11:04 浏览: 29
(1) 在Python中创建一个长度为n的单链表通常涉及定义一个节点类(Node)和一个链表类(LinkedList),步骤如下:
```python
class Node:
def __init__(self, data=None):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
```
这里初始化了一个空链表,`head`指向None。
(2) 插入新节点到链表的操作可以添加在`LinkedList`类中,例如在头部插入:
```python
def insert_at_start(self, data):
new_node = Node(data)
new_node.next = self.head
self.head = new_node
```
如果要在特定位置插入,需要遍历找到该位置。
(3) 删除某个节点需要遍历查找目标节点,并处理前驱节点的链接。删除指定数据的节点示例:
```python
def delete_node(self, value):
if self.head is not None and self.head.data == value:
self.head = self.head.next
return
current = self.head
while current.next is not None:
if current.next.data == value:
current.next = current.next.next
break
current = current.next
```
(4) 打印输出链表中的所有节点元素值:
```python
def print_list(self):
current = self.head
while current is not None:
print(current.data, end=" -> ")
current = current.next
print("None")
```
阅读全文
相关推荐
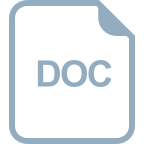
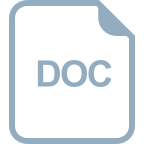
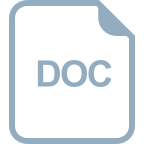










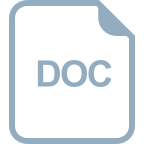
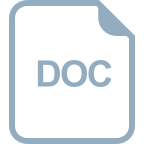
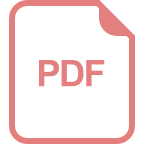
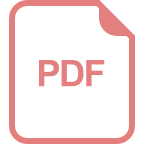