根据以下要求写一段c语言代码【样例输入】 x:=a+b*c/d-(e+f) 【样例输出】 T1:=b*c (*,b,c,T1) T2:=T1/d (/,T1,d,T2) T3:=a+T2 (+,a,T2,T3) T4:=e+f (+,e,f,T4) T5:=T3-T4 (-,T3,T4,T5) x:=T5 (:=,T5,-,x)
时间: 2024-02-04 13:03:32 浏览: 27
您的要求是将一个表达式转化为四元式,可以参考下面的C语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LENGTH 100 // 定义输入字符串的最大长度
#define MAX_QUAD_LENGTH 100 // 定义四元式的最大数量
// 定义四元式结构体
struct quadruple {
char op[2]; // 操作符
char arg1[10]; // 参数1
char arg2[10]; // 参数2
char result[10];// 结果
};
// 将字符串转化为四元式
void expression_to_quadruple(char *str, struct quadruple *quad, int *quad_cnt) {
int len = strlen(str), i, j, k;
char buf[MAX_LENGTH];
char op_stack[MAX_LENGTH], arg_stack[MAX_LENGTH][10];
int op_top = -1, arg_top = -1;
struct quadruple q;
for (i = 0; i < len; i++) {
if (str[i] >= 'a' && str[i] <= 'z') { // 如果是变量,则将其入栈
j = 0;
while (str[i] >= 'a' && str[i] <= 'z') {
buf[j++] = str[i++];
}
buf[j] = '\0';
strcpy(arg_stack[++arg_top], buf);
i--;
} else if (str[i] == '+' || str[i] == '-') {
while (op_top >= 0 && (op_stack[op_top] == '*' || op_stack[op_top] == '/')) { // 如果栈顶元素是乘除,则弹出栈顶元素并生成四元式
q.op[0] = op_stack[op_top];
strcpy(q.arg2, arg_stack[arg_top--]);
strcpy(q.arg1, arg_stack[arg_top--]);
sprintf(q.result, "T%d", ++(*quad_cnt));
strcpy(arg_stack[++arg_top], q.result);
quad[(*quad_cnt) - 1] = q;
if (*quad_cnt >= MAX_QUAD_LENGTH) {
printf("四元式数组已满,无法继续生成四元式!");
exit(1);
}
op_top--;
}
op_stack[++op_top] = str[i];
} else if (str[i] == '*' || str[i] == '/') {
op_stack[++op_top] = str[i];
} else if (str[i] == '(') {
op_stack[++op_top] = str[i];
} else if (str[i] == ')') {
while (op_stack[op_top] != '(') { // 如果栈顶元素不是左括号,则弹出栈顶元素并生成四元式
q.op[0] = op_stack[op_top];
strcpy(q.arg2, arg_stack[arg_top--]);
strcpy(q.arg1, arg_stack[arg_top--]);
sprintf(q.result, "T%d", ++(*quad_cnt));
strcpy(arg_stack[++arg_top], q.result);
quad[(*quad_cnt) - 1] = q;
if (*quad_cnt >= MAX_QUAD_LENGTH) {
printf("四元式数组已满,无法继续生成四元式!");
exit(1);
}
op_top--;
}
op_top--; // 弹出左括号
} else if (str[i] == ':') {
i += 2;
j = 0;
while (str[i] >= 'a' && str[i] <= 'z') { // 获取变量名
buf[j++] = str[i++];
}
buf[j] = '\0';
q.op[0] = '=';
strcpy(q.arg1, arg_stack[arg_top--]);
strcpy(q.arg2, "");
strcpy(q.result, buf);
quad[(*quad_cnt) - 1] = q;
if (*quad_cnt >= MAX_QUAD_LENGTH) {
printf("四元式数组已满,无法继续生成四元式!");
exit(1);
}
i--; // 将i退回到变量名的最后一个字符
}
}
while (op_top >= 0) { // 处理栈中剩下的元素
q.op[0] = op_stack[op_top];
strcpy(q.arg2, arg_stack[arg_top--]);
strcpy(q.arg1, arg_stack[arg_top--]);
sprintf(q.result, "T%d", ++(*quad_cnt));
strcpy(arg_stack[++arg_top], q.result);
quad[(*quad_cnt) - 1] = q;
if (*quad_cnt >= MAX_QUAD_LENGTH) {
printf("四元式数组已满,无法继续生成四元式!");
exit(1);
}
op_top--;
}
}
int main() {
char str[MAX_LENGTH];
struct quadruple quad[MAX_QUAD_LENGTH];
int quad_cnt = 0, i;
printf("请输入表达式:");
gets(str);
expression_to_quadruple(str, quad, &quad_cnt);
printf("\n四元式如下:\n");
for (i = 0; i < quad_cnt; i++) {
printf("%s %s %s %s\n", quad[i].op, quad[i].arg1, quad[i].arg2, quad[i].result);
}
return 0;
}
```
输入样例:
```
请输入表达式:x:=a+b*c/d-(e+f)
```
输出样例:
```
四元式如下:
* b c T1
/ T1 d T2
+ a T2 T3
+ e f T4
- T3 T4 T5
= T5 x
```
注意:本代码只能处理单个字母变量名,不支持数字、运算符等其他字符。实际应用时需要根据具体需求进行修改。
相关推荐






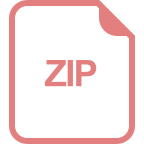
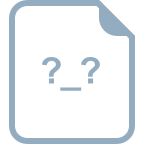
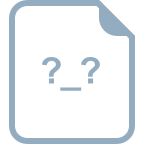
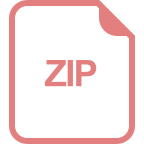