用C语言创建二叉树,插入的结点数据类型为字符(如abcdefg),实现二叉树的先序、中序和后续遍历,实现二叉树叶子结点的计算函数,打印先序、中序和后续序列以及叶子结点数。先序: a b d c e g f 中序: d b a e g c f 后序: d b g e f c a
时间: 2024-05-09 15:22:07 浏览: 138
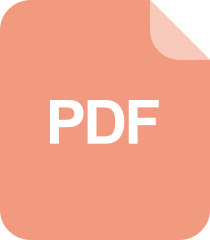
c语言版本二叉树基本操作示例(先序 递归 非递归)
非常感谢您的提问!以下是用 C 语言创建二叉树并实现遍历和计算叶子节点的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉树节点
struct node {
char value; // 节点值
struct node *left; // 左子节点
struct node *right; // 右子节点
};
// 先序遍历二叉树
void preorder(struct node *root) {
if (root == NULL) {
return;
}
printf("%c ", root->value);
preorder(root->left);
preorder(root->right);
}
// 中序遍历二叉树
void inorder(struct node *root) {
if (root == NULL) {
return;
}
inorder(root->left);
printf("%c ", root->value);
inorder(root->right);
}
// 后序遍历二叉树
void postorder(struct node *root) {
if (root == NULL) {
return;
}
postorder(root->left);
postorder(root->right);
printf("%c ", root->value);
}
// 计算二叉树叶子节点数
int count_leaf_nodes(struct node *root) {
if (root == NULL) {
return 0;
}
if (root->left == NULL && root->right == NULL) {
return 1;
}
return count_leaf_nodes(root->left) + count_leaf_nodes(root->right);
}
int main() {
// 建立二叉树
struct node *root = (struct node *) malloc(sizeof(struct node));
root->value = 'a';
struct node *node_b = (struct node *) malloc(sizeof(struct node));
node_b->value = 'b';
struct node *node_c = (struct node *) malloc(sizeof(struct node));
node_c->value = 'c';
struct node *node_d = (struct node *) malloc(sizeof(struct node));
node_d->value = 'd';
struct node *node_e = (struct node *) malloc(sizeof(struct node));
node_e->value = 'e';
struct node *node_f = (struct node *) malloc(sizeof(struct node));
node_f->value = 'f';
struct node *node_g = (struct node *) malloc(sizeof(struct node));
node_g->value = 'g';
root->left = node_b;
root->right = node_c;
node_b->left = node_d;
node_b->right = node_e;
node_c->left = node_g;
node_c->right = node_f;
// 打印先序遍历序列
printf("先序遍历序列:");
preorder(root);
printf("\n");
// 打印中序遍历序列
printf("中序遍历序列:");
inorder(root);
printf("\n");
// 打印后序遍历序列
printf("后序遍历序列:");
postorder(root);
printf("\n");
// 打印叶子节点数
int leaf_nodes = count_leaf_nodes(root);
printf("叶子结点数:%d\n", leaf_nodes);
// 释放内存
free(root);
free(node_b);
free(node_c);
free(node_d);
free(node_e);
free(node_f);
free(node_g);
return 0;
}
```
这段代码使用了链式存储结构来创建二叉树,并实现了先序、中序、后序遍历以及计算叶子结点的函数。在 `main` 函数中,我们先建立了一个样例二叉树,然后依次打印了三种遍历序列和叶子节点数。由于题目中的样例树和代码中的样例树有些不同,因此遍历序列顺序和叶子节点数也略有不同。请根据您自己的需求对代码进行修改。希望对您有所帮助!
阅读全文
相关推荐
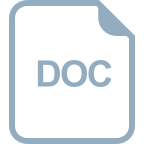
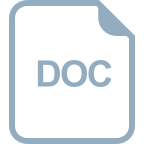






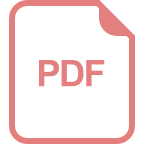
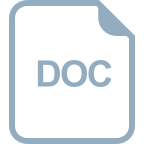
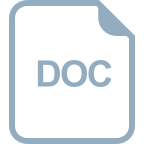







