js文件中定义了几个相互关联的方法,vue中调用
时间: 2023-08-16 09:05:57 浏览: 45
在Vue中调用一个js文件中定义的相互关联的方法,可以先将该js文件引入到Vue组件中,然后在Vue组件的methods中定义一个方法,该方法通过调用引入的js文件中的方法实现相应的功能。
示例代码如下:
```html
<template>
<div>
<button @click="handleClick">点击</button>
</div>
</template>
<script>
import { func1, func2 } from './utils.js';
export default {
name: 'MyComponent',
methods: {
handleClick() {
// 调用 utils.js 中的方法
const result1 = func1();
const result2 = func2(result1);
console.log(result2);
}
}
}
</script>
```
其中,`utils.js`文件中定义了`func1`和`func2`两个方法,`func2`方法依赖于`func1`方法的返回值。在Vue组件中引入`utils.js`文件,并在`handleClick`方法中调用`func1`和`func2`方法,从而实现相应的功能。
相关问题
如何在vue中调用js文件中定义的event
在 Vue 中调用 js 文件中定义的 event,可以通过以下几个步骤:
1.创建一个事件总线(Event Bus)
```
import Vue from 'vue'
export const EventBus = new Vue()
```
2.在 js 文件中触发事件
```
import {EventBus} from './event-bus.js'
EventBus.$emit('my-event', data)
```
3.在 Vue 组件中监听事件
```
import {EventBus} from './event-bus.js'
export default {
created() {
// 监听 my-event 事件
EventBus.$on('my-event', this.handleMyEvent)
},
methods: {
handleMyEvent(data) {
// 处理事件
}
}
}
```
这样,当在 js 文件中触发 'my-event' 事件时,Vue 组件中定义的 handleMyEvent 方法就会被调用。
vue中定义多个实例相互调用
在 Vue 中,你可以通过在组件中使用 `$refs` 属性来获取其他组件的实例,并调用其方法或访问其属性。
例如,假设你有两个组件 `componentA` 和 `componentB`,你可以在 `componentA` 中使用 `$refs` 来获取 `componentB` 的实例,并调用其方法:
```html
<template>
<div>
<component-b ref="componentB"></component-b>
<button @click="callComponentBMethod">Call Component B Method</button>
</div>
</template>
<script>
import ComponentB from './ComponentB.vue'
export default {
components: {
ComponentB
},
methods: {
callComponentBMethod() {
this.$refs.componentB.componentBMethod()
}
}
}
</script>
```
在上面的示例中,我们在 `componentA` 中定义了一个名称为 `componentB` 的 `$refs`,然后在 `callComponentBMethod` 方法中使用 `$refs` 来获取 `componentB` 的实例,并调用其 `componentBMethod` 方法。
需要注意的是,在获取 `$refs` 时,你需要等到组件已经被挂载到 DOM 上才能获取到其实例。因此,如果你想在组件的 `mounted` 生命周期钩子中获取 `$refs`,你需要使用 `this.$nextTick` 方法来等待 DOM 更新完成。
另外,如果你需要在多个组件之间共享数据或状态,你可以考虑使用 Vuex 进行全局状态管理。
相关推荐
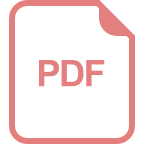
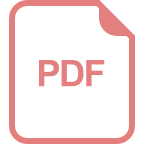












