golang resample timeseries data
时间: 2023-08-06 16:07:42 浏览: 47
在 Golang 中,你可以使用第三方库来对时间序列数据进行重采样。以下是使用 gonum/series 和 gonum/stat 库的示例代码:
```go
package main
import (
"fmt"
"time"
"gonum.org/v1/gonum/floats"
"gonum.org/v1/gonum/series"
"gonum.org/v1/gonum/stat"
)
func main() {
// 创建原始时间序列数据
times := []time.Time{
time.Date(2021, 1, 1, 0, 0, 0, 0, time.UTC),
time.Date(2021, 1, 2, 0, 0, 0, 0, time.UTC),
time.Date(2021, 1, 3, 0, 0, 0, 0, time.UTC),
time.Date(2021, 1, 4, 0, 0, 0, 0, time.UTC),
time.Date(2021, 1, 5, 0, 0, 0, 0, time.UTC),
}
values := []float64{1, 2, 3, 4, 5}
ts := series.New(times, values, nil)
// 重采样为每天的平均值
resampled, err := ts.Resample(&time.Duration{24 * time.Hour}, series.Mean)
if err != nil {
panic(err)
}
// 输出重采样后的时间序列数据
fmt.Println("Resampled times:")
for _, t := range resampled.Times() {
fmt.Println(t)
}
fmt.Println("Resampled values:")
fmt.Println(resampled.Values())
// 计算重采样后的时间序列数据的均值
mean := stat.Mean(resampled.Values(), nil)
fmt.Println("Resampled mean:", mean)
}
```
在上面的示例代码中,我们使用了 `gonum/series` 库来创建原始的时间序列数据。然后,我们使用 `Resample()` 函数来对时间序列数据进行重采样,将原始数据重采样为每天的平均值。最后,我们使用 `gonum/stat` 库来计算重采样后的时间序列数据的均值。
请注意,我们使用了 `time.Duration` 类型来指定重采样时间间隔。在本例中,我们将其设置为 24 小时,因此重采样后的数据将按天进行分组。你可以根据自己的需求调整这个时间间隔。
相关推荐
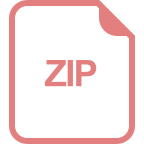
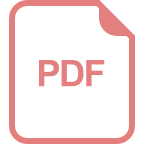














