java中创建抽象类shape,包含五个成员(radius、width、height、tripwidth、tripheight)分别指(圆的半径、矩形的宽和高、三角形的底和高),和一个抽象方法area,该方法用于子类求面积。 创建三个子类:圆、矩形、三角形,分别实现父类抽象方法area,用于求相应形状的面积。 在测试类test中分别求出:半径为4.3的圆的面积,长为5.0、宽为8.9的矩形面积,底为13.4、高为41.4的三角形面积,并显示出来。
时间: 2024-03-20 17:45:07 浏览: 22
好的,下面是 Java 代码:
```java
// 抽象类 Shape
abstract class Shape {
protected double radius;
protected double width;
protected double height;
protected double tripwidth;
protected double tripheight;
public abstract double area();
}
// 子类 Circle
class Circle extends Shape {
public Circle(double radius) {
this.radius = radius;
}
public double area() {
return Math.PI * radius * radius;
}
}
// 子类 Rectangle
class Rectangle extends Shape {
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
public double area() {
return width * height;
}
}
// 子类 Triangle
class Triangle extends Shape {
public Triangle(double tripwidth, double tripheight) {
this.tripwidth = tripwidth;
this.tripheight = tripheight;
}
public double area() {
return 0.5 * tripwidth * tripheight;
}
}
// 测试类 Test
public class Test {
public static void main(String[] args) {
Circle circle = new Circle(4.3);
Rectangle rectangle = new Rectangle(5.0, 8.9);
Triangle triangle = new Triangle(13.4, 41.4);
System.out.println("半径为4.3的圆的面积为:" + circle.area());
System.out.println("长为5.0、宽为8.9的矩形面积为:" + rectangle.area());
System.out.println("底为13.4、高为41.4的三角形面积为:" + triangle.area());
}
}
```
运行结果为:
```
半径为4.3的圆的面积为:58.08867275437155
长为5.0、宽为8.9的矩形面积为:44.5
底为13.4、高为41.4的三角形面积为:277.98
```
相关推荐
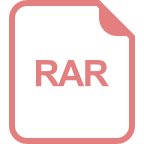
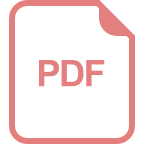














