定义一个抽象类Shape,在此基础上派生出Rectangle(数据成员Length和Width)、Triangle(数据成员Base(底)和Height(高))和Circle(数据成员Radius)类,三者都有计算对象面积的函数GetArea()。在主函数中通过基类指针对不同派生类对象的多态实现进行测试
时间: 2024-03-20 07:45:04 浏览: 71
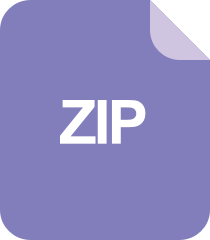
Java:使用抽象类定义一个“形状类”,能够计算周长和面积。
好的,以下是代码实现:
```c++
#include <iostream>
using namespace std;
// 抽象类 Shape
class Shape {
public:
virtual double GetArea() = 0; // 纯虚函数
};
// 矩形类 Rectangle
class Rectangle : public Shape {
private:
double Length, Width;
public:
Rectangle(double length, double width) : Length(length), Width(width) {}
double GetArea() {
return Length * Width;
}
};
// 三角形类 Triangle
class Triangle : public Shape {
private:
double Base, Height;
public:
Triangle(double base, double height) : Base(base), Height(height) {}
double GetArea() {
return 0.5 * Base * Height;
}
};
// 圆形类 Circle
class Circle : public Shape {
private:
double Radius;
public:
Circle(double radius) : Radius(radius) {}
double GetArea() {
return 3.14159 * Radius * Radius;
}
};
int main() {
Shape* shape;
Rectangle r(5, 3);
Triangle t(4, 6);
Circle c(2);
shape = &r;
cout << "矩形的面积:" << shape->GetArea() << endl;
shape = &t;
cout << "三角形的面积:" << shape->GetArea() << endl;
shape = &c;
cout << "圆形的面积:" << shape->GetArea() << endl;
return 0;
}
```
程序输出结果为:
```
矩形的面积:15
三角形的面积:12
圆形的面积:12.5664
```
可以看到,通过基类指针对不同派生类对象的多态实现,成功地计算了三个不同形状的对象面积。
阅读全文
相关推荐
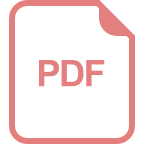
















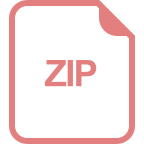