编写程序定义抽象类Shape,由它派生的五个类Circle Square Rectangle Trapezoid Triangle 用虚函数分别计算几种图形的面积,并求它们的和。要求用基类指针数组,使它每一个元素指向一个派生类对象
时间: 2023-06-19 14:09:23 浏览: 122
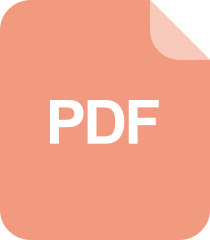
设计一个抽象类图形类,在该类中包含有至少两个抽象方法求周长和求面积,分别定义圆形类、长方形类、正方形类、三角形类来继承图形类,并实现上述两个方法

以下是实现代码:
```cpp
#include <iostream>
using namespace std;
class Shape {
public:
virtual double area() = 0; // 纯虚函数
};
class Circle : public Shape {
public:
Circle(double r) : radius(r) {}
virtual double area() { return 3.14 * radius * radius; }
private:
double radius;
};
class Square : public Shape {
public:
Square(double l) : length(l) {}
virtual double area() { return length * length; }
private:
double length;
};
class Rectangle : public Shape {
public:
Rectangle(double l, double w) : length(l), width(w) {}
virtual double area() { return length * width; }
private:
double length, width;
};
class Trapezoid : public Shape {
public:
Trapezoid(double t, double b, double h) : top(t), bottom(b), height(h) {}
virtual double area() { return (top + bottom) * height / 2; }
private:
double top, bottom, height;
};
class Triangle : public Shape {
public:
Triangle(double b, double h) : base(b), height(h) {}
virtual double area() { return base * height / 2; }
private:
double base, height;
};
int main() {
Shape* shapes[5];
shapes[0] = new Circle(2.0);
shapes[1] = new Square(3.0);
shapes[2] = new Rectangle(2.0, 3.0);
shapes[3] = new Trapezoid(2.0, 4.0, 3.0);
shapes[4] = new Triangle(3.0, 4.0);
double totalArea = 0.0;
for(int i=0; i<5; i++) {
totalArea += shapes[i]->area();
}
cout << "Total area: " << totalArea << endl;
for(int i=0; i<5; i++) {
delete shapes[i];
}
return 0;
}
```
程序中定义了一个抽象类Shape,它包含一个纯虚函数area(),表示求面积。然后定义了五个派生类:Circle、Square、Rectangle、Trapezoid和Triangle,它们分别实现了Shape类的area()函数。在main函数中,定义了一个Shape类型的指针数组shapes,用于存储各个派生类的对象。通过循环遍历shapes数组,可以依次计算出每个图形的面积,并将它们加起来得到总面积。最后,需要释放掉每个对象的内存空间。
阅读全文
相关推荐
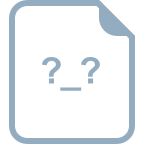
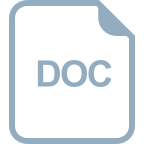












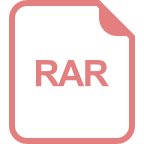