用C++编写一段简单完整的程序,定义抽象类Shape,由它派生出5个派生类Circle(圆形)、Square(正方形)Rectangle (矩形)、Trapezoid(梯形)、Triangle(三角形)。用虚函数分别计算几种图形的面积,并求它们的和。要求:用基类指针数组,使它每一个元素指向一个派生类对象
时间: 2024-02-26 14:56:24 浏览: 24
以下是一段简单的程序,实现了上述要求:
```c++
#include <iostream>
#include <cmath>
using namespace std;
class Shape {
public:
virtual double area() = 0;
};
class Circle : public Shape {
public:
Circle(double r) : radius(r) {}
double area() {
return M_PI * radius * radius;
}
private:
double radius;
};
class Square : public Shape {
public:
Square(double l) : length(l) {}
double area() {
return length * length;
}
private:
double length;
};
class Rectangle : public Shape {
public:
Rectangle(double l, double w) : length(l), width(w) {}
double area() {
return length * width;
}
private:
double length;
double width;
};
class Trapezoid : public Shape {
public:
Trapezoid(double b1, double b2, double h) : base1(b1), base2(b2), height(h) {}
double area() {
return (base1 + base2) * height / 2;
}
private:
double base1;
double base2;
double height;
};
class Triangle : public Shape {
public:
Triangle(double b, double h) : base(b), height(h) {}
double area() {
return base * height / 2;
}
private:
double base;
double height;
};
int main() {
Shape* shapes[5];
shapes[0] = new Circle(5);
shapes[1] = new Square(4);
shapes[2] = new Rectangle(3, 5);
shapes[3] = new Trapezoid(2, 5, 3);
shapes[4] = new Triangle(4, 3);
double sum = 0;
for (int i = 0; i < 5; i++) {
sum += shapes[i]->area();
}
cout << "Total area: " << sum << endl;
for (int i = 0; i < 5; i++) {
delete shapes[i];
}
return 0;
}
```
程序中,我们定义了一个抽象类 `Shape`,它包含了一个纯虚函数 `area()`,用于计算图形的面积。然后,我们定义了 5 个派生类 `Circle`、`Square`、`Rectangle`、`Trapezoid` 和 `Triangle`,分别计算它们的面积。在 `main()` 函数中,我们用基类指针数组 `shapes` 存储这 5 种派生类的对象,并分别调用它们的 `area()` 函数计算面积。最后,我们将每个图形的面积相加,得到了它们的总面积。
注意,我们在程序结束之前需要删除 `shapes` 数组中的每个对象,以避免内存泄漏。
相关推荐
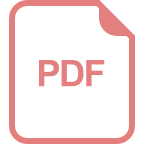
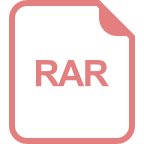
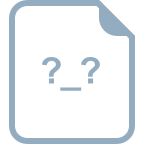














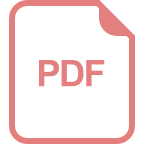