编写一个程序,定义抽象基类Shape,由它派生出5个派生类:Circle、Square、Rectangle、Trapezoid、Triangle。用虛函数分别计算几种图形面积,并求它们之和
时间: 2023-07-10 10:32:02 浏览: 96
好的,这是一个经典的面向对象程序设计问题。以下是一个示例代码:
```c++
#include <iostream>
#include <vector>
class Shape {
public:
virtual double area() const = 0; // 纯虚函数
};
class Circle : public Shape {
public:
Circle(double r) : radius(r) {}
double area() const override {
return 3.14159 * radius * radius;
}
private:
double radius;
};
class Square : public Shape {
public:
Square(double s) : side(s) {}
double area() const override {
return side * side;
}
private:
double side;
};
class Rectangle : public Shape {
public:
Rectangle(double w, double h) : width(w), height(h) {}
double area() const override {
return width * height;
}
private:
double width, height;
};
class Trapezoid : public Shape {
public:
Trapezoid(double a, double b, double h) : top(a), bottom(b), height(h) {}
double area() const override {
return (top + bottom) * height / 2;
}
private:
double top, bottom, height;
};
class Triangle : public Shape {
public:
Triangle(double b, double h) : base(b), height(h) {}
double area() const override {
return base * height / 2;
}
private:
double base, height;
};
int main() {
std::vector<Shape*> shapes;
shapes.push_back(new Circle(2.0));
shapes.push_back(new Square(3.0));
shapes.push_back(new Rectangle(2.0, 3.0));
shapes.push_back(new Trapezoid(2.0, 4.0, 3.0));
shapes.push_back(new Triangle(2.0, 3.0));
double total_area = 0.0;
for (auto shape : shapes) {
total_area += shape->area();
}
std::cout << "Total area: " << total_area << std::endl;
for (auto shape : shapes) {
delete shape;
}
return 0;
}
```
这个程序定义了一个抽象基类 Shape,它有一个纯虚函数 area(),因此不能被实例化。然后它派生出了五个具体的形状类,并实现了它们的 area() 函数。在主函数中,我们创建了一个 Shape 指针的 vector,向其中添加了五个具体形状的实例。然后通过循环计算它们的面积,并输出总面积。最后需要释放内存,避免内存泄漏。
阅读全文
相关推荐
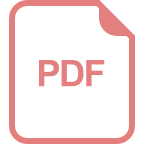
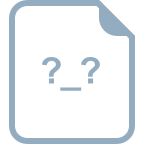












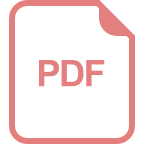
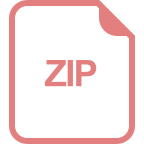
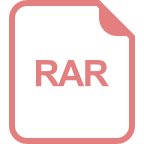