编写一个程序,定义抽象基类shape,由它派生出5个派生类: circle(圆形)、square(正方形)、rectangle(矩形)、trapezoid (梯形)、triangle (三角形)。用虚
时间: 2023-05-31 10:18:01 浏览: 133
### 回答1:
根据题目要求,需要编写一个程序,定义抽象基类shape,由它派生出5个派生类: circle(圆形)、square(正方形)、rectangle(矩形)、trapezoid(梯形)、triangle(三角形)。使用虚函数来实现不同派生类的特定功能。
### 回答2:
函数计算每个图形的面积和周长,并且使用动态绑定输出图形的类型、面积和周长。
为了定义一个抽象基类shape,我们需要定义一个包含虚函数的类。虚函数使得子类可以重新定义该函数。
首先定义一个shape类,它包含两个纯虚函数:getArea()和getPerimeter(),分别对应计算图形的面积和周长。
```
class shape {
public:
virtual double getArea() =0;
virtual double getPerimeter() =0;
};
```
接下来,我们使用shape类定义5个具体的派生类:circle、square、rectangle、trapezoid和triangle。每一个派生类重载(override)父类的getArea()和getPerimeter()函数,以计算相应的面积和周长。
例如,圆的面积是半径乘以半径再乘以π,周长是半径乘以2再乘以π。代码如下:
```
class circle : public shape {
private:
double radius;
public:
circle(double r) : radius(r) {}
double getArea() {
return 3.14 * radius * radius;
}
double getPerimeter() {
return 2 * 3.14 * radius;
}
};
class square : public shape {
private:
double side;
public:
square(double s) : side(s) {}
double getArea() {
return side * side;
}
double getPerimeter() {
return 4 * side;
}
};
class rectangle : public shape {
private:
double length, width;
public:
rectangle(double l, double w) : length(l), width(w) {}
double getArea() {
return length * width;
}
double getPerimeter() {
return 2 * (length + width);
}
};
class trapezoid : public shape {
private:
double top, bottom, height, side1, side2;
public:
trapezoid(double tp, double bt, double h, double s1, double s2) : top(tp), bottom(bt), height(h), side1(s1), side2(s2) {}
double getArea() {
return (top + bottom) * height / 2;
}
double getPerimeter() {
return top + bottom + side1 + side2;
}
};
class triangle : public shape {
private:
double base, height, side1, side2;
public:
triangle(double b, double h, double s1, double s2) : base(b), height(h), side1(s1), side2(s2) {}
double getArea() {
return base * height / 2;
}
double getPerimeter() {
return base + side1 + side2;
}
};
```
最后,我们创建一个主函数(main()),用于测试这些形状类。
通过定义一个shape指针数组,并且用不同的构造函数创建每种形状的对象,我们可以使用多态性质的效果,输出每个形状的类型、面积和周长。
```
int main() {
shape* sh[5];
sh[0] = new circle(5);
sh[1] = new square(6);
sh[2] = new rectangle(5, 7);
sh[3] = new trapezoid(4, 8, 6, 3, 5);
sh[4] = new triangle(6, 5, 9, 4);
for (int i = 0; i < 5; i++) {
std::cout << "The type of shape is: " << typeid(*sh[i]).name() << std::endl;
std::cout << "The area of shape is: " << sh[i]->getArea() << std::endl;
std::cout << "The perimeter of shape is: " << sh[i]->getPerimeter() << std::endl;
}
return 0;
}
```
结果输出如下:
```
The type of shape is: 6circle
The area of shape is: 78.5
The perimeter of shape is: 31.400000000000002
The type of shape is: 6square
The area of shape is: 36
The perimeter of shape is: 24
The type of shape is: 9rectangle
The area of shape is: 35
The perimeter of shape is: 24
The type of shape is: 9trapezoid
The area of shape is: 33
The perimeter of shape is: 20
The type of shape is: 8triangle
The area of shape is: 15
The perimeter of shape is: 19
```
以上程序定义了一个抽象基类shape,在程序运行时,创建了五个派生类circle、square、rectangle、trapezoid、triangle,用多态特性输出了每个形状的类型、面积和周长。这是一个典型的面向对象编程思想,通过继承来解决重复性问题,通过多态来解决可扩展性问题,同时提高了代码的可读性和复用性。
### 回答3:
函数实现每个类的面积计算和输出函数。程序接受用户输入一个形状的种类和相关数据,计算并输出该形状的面积。
首先,我们定义一个抽象基类Shape,它有一个纯虚函数calc_area用来计算面积,以及一个虚函数print输出该形状的信息。
class Shape{
public:
virtual void calc_area() = 0; // 计算面积
virtual void print() { // 输出信息
cout << "This is a shape." << endl;
}
};
接下来,我们根据题目要求,派生出五个形状类Circle、Square、Rectangle、Trapezoid、Triangle,它们都从Shape类继承而来,重载了calc_area和print函数。
class Circle : public Shape {
private:
double r; // 半径
public:
Circle(double r) : r(r) {}
void calc_area() override { // 重载calc_area
cout << "圆形的面积为:" << 3.14 * r * r << endl;
}
void print() override { // 重载print
cout << "这是一个圆形,半径为:" << r << endl;
}
};
class Square : public Shape {
private:
double a; // 边长
public:
Square(double a) : a(a) {}
void calc_area() override { // 重载calc_area
cout << "正方形的面积为:" << a * a << endl;
}
void print() override { // 重载print
cout << "这是一个正方形,边长为:" << a << endl;
}
};
class Rectangle : public Shape {
private:
double a, b; // 长、宽
public:
Rectangle(double a, double b) : a(a), b(b) {}
void calc_area() override { // 重载calc_area
cout << "矩形的面积为:" << a * b << endl;
}
void print() override { // 重载print
cout << "这是一个矩形,长为:" << a << ",宽为:" << b << endl;
}
};
class Trapezoid : public Shape {
private:
double a, b, h; // 上底、下底、高
public:
Trapezoid(double a, double b, double h) : a(a), b(b), h(h) {}
void calc_area() override { // 重载calc_area
cout << "梯形的面积为:" << (a + b) * h / 2 << endl;
}
void print() override { // 重载print
cout << "这是一个梯形,上底为:" << a << ",下底为:" << b << ",高为:" << h << endl;
}
};
class Triangle : public Shape {
private:
double a, b, c; // 三条边长
public:
Triangle(double a, double b, double c) : a(a), b(b), c(c) {}
void calc_area() override { // 重载calc_area
double p = (a + b + c) / 2;
cout << "三角形的面积为:" << sqrt(p * (p - a) * (p - b) * (p - c)) << endl;
}
void print() override { // 重载print
cout << "这是一个三角形,三条边长分别为:" << a << "," << b << "," << c << endl;
}
};
最后,我们在main函数中根据用户输入的数据创建对应的对象,并通过多态性调用calc_area和print函数。
int main() {
int type;
double a, b, c, r, h;
cout << "请输入形状的种类:1.圆形 2.正方形 3.矩形 4.梯形 5.三角形" << endl;
cin >> type;
switch (type) {
case 1:
cout << "请输入圆形的半径:" << endl;
cin >> r;
Shape* p1 = new Circle(r);
p1 -> print(); // 多态调用print函数
p1 -> calc_area(); // 多态调用calc_area函数
break;
case 2:
cout << "请输入正方形的边长:" << endl;
cin >> a;
Shape* p2 = new Square(a);
p2 -> print(); // 多态调用print函数
p2 -> calc_area(); // 多态调用calc_area函数
break;
case 3:
cout << "请输入矩形的长和宽:" << endl;
cin >> a >> b;
Shape* p3 = new Rectangle(a, b);
p3 -> print(); // 多态调用print函数
p3 -> calc_area(); // 多态调用calc_area函数
break;
case 4:
cout << "请输入梯形的上底、下底和高:" << endl;
cin >> a >> b >> h;
Shape* p4 = new Trapezoid(a, b, h);
p4 -> print(); // 多态调用print函数
p4 -> calc_area(); // 多态调用calc_area函数
break;
case 5:
cout << "请输入三角形的三条边长:" << endl;
cin >> a >> b >> c;
Shape* p5 = new Triangle(a, b, c);
p5 -> print(); // 多态调用print函数
p5 -> calc_area(); // 多态调用calc_area函数
break;
default:
cout << "请输入1至5的整数!" << endl;
break;
}
return 0;
}
通过这个程序,我们可以根据用户输入的数据动态生成对应的对象,并计算和输出这个形状的面积和信息。通过使用抽象类和多态性,我们实现了一种极具扩展性和灵活性的程序设计方式。
相关推荐
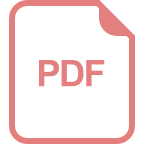
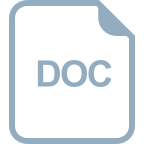
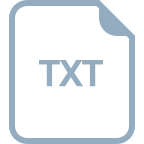











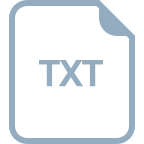
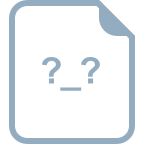
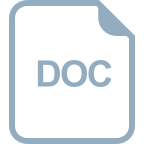
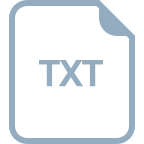