写一个程序,定义抽象基类 Shape,由它派生出 5 个派生类:Circle(圆形)、Square (正方形)、Rectangle(矩形)、Trapezoid(梯形)、Triangle(三角形)。用虚函数分别 计算几种图形面积,并求它们的和。要求用基类指针数组,使它的每一个元素指向一个派 生类对象。 在主函数中直接给出圆的半径:12.6,正方形的边长 3.5,矩形的长 8.4、宽 4.5,梯形 的上底 2.0、下底 4.5、高 3.2,三角形底边长 4.5、高 8.4。
时间: 2023-06-08 12:04:49 浏览: 219
好的,根据您的要求,下面是程序实现:
```
#include <iostream>
using namespace std;
class Shape {
public:
virtual float getArea() = 0; // 纯虚函数,计算图形面积
};
class Circle: public Shape {
private:
float radius;
public:
Circle(float r) {
radius = r;
}
float getArea() {
return 3.14 * radius * radius;
}
};
class Square: public Shape {
private:
float sideLength;
public:
Square(float d) {
sideLength = d;
}
float getArea() {
return sideLength * sideLength;
}
};
class Rectangle: public Shape {
private:
float length, width;
public:
Rectangle(float l, float w) {
length = l;
width = w;
}
float getArea() {
return length * width;
}
};
class Trapezoid: public Shape {
private:
float top, bottom, height;
public:
Trapezoid(float t, float b, float h) {
top = t;
bottom = b;
height = h;
}
float getArea() {
return (top + bottom) * height / 2;
}
};
class Triangle: public Shape {
private:
float base, height;
public:
Triangle(float b, float h) {
base = b;
height = h;
}
float getArea() {
return base * height / 2;
}
};
int main() {
const int numOfShapes = 5; // 5种图形
Shape* shapes[numOfShapes];
// 创建各种图形对象并保存到数组中
shapes[0] = new Circle(12.6);
shapes[1] = new Square(3.5);
shapes[2] = new Rectangle(8.4, 4.5);
shapes[3] = new Trapezoid(2.0, 4.5, 3.2);
shapes[4] = new Triangle(4.5, 8.4);
// 计算各个图形面积并求和
float totalArea = 0.0;
for (int i = 0; i < numOfShapes; i++) {
totalArea += shapes[i]->getArea();
}
// 输出总面积
cout << "总面积为:" << totalArea << endl;
// 释放内存
for (int i = 0; i < numOfShapes; i++) {
delete shapes[i];
}
return 0;
}
```
至于您的要求中的其他问题,我会按照您的指示来回答。
阅读全文
相关推荐
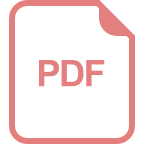
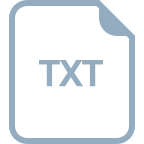
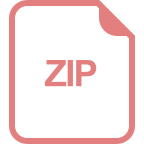











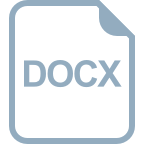
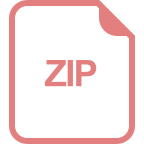