复数四则运算使用类与对象
时间: 2023-06-16 18:03:15 浏览: 70
复数可以表示为 a+bi 的形式,其中 a 和 b 分别为实部和虚部,i 表示虚数单位。在类中可以定义实部和虚部作为类的成员变量,然后实现加、减、乘、除四种运算的成员函数。
下面是一个示例代码:
```cpp
#include <iostream>
using namespace std;
class Complex {
private:
double real; // 实部
double imag; // 虚部
public:
Complex(double r = 0, double i = 0) {
real = r;
imag = i;
}
Complex operator+(const Complex& other) const {
return Complex(real + other.real, imag + other.imag);
}
Complex operator-(const Complex& other) const {
return Complex(real - other.real, imag - other.imag);
}
Complex operator*(const Complex& other) const {
return Complex(real * other.real - imag * other.imag, real * other.imag + imag * other.real);
}
Complex operator/(const Complex& other) const {
double denominator = other.real * other.real + other.imag * other.imag;
return Complex((real * other.real + imag * other.imag) / denominator, (imag * other.real - real * other.imag) / denominator);
}
void print() const {
cout << real << "+" << imag << "i" << endl;
}
};
int main() {
Complex a(1, 2);
Complex b(3, 4);
Complex c = a + b;
c.print(); // 输出 4+6i
Complex d = a - b;
d.print(); // 输出 -2-2i
Complex e = a * b;
e.print(); // 输出 -5+10i
Complex f = a / b;
f.print(); // 输出 0.44+0.08i
return 0;
}
```
在上面的代码中,定义了一个 Complex 类,其中包含实部和虚部两个成员变量。四个运算符重载函数分别实现了加、减、乘、除四种运算,并返回一个新的 Complex 对象。print 函数用于输出 Complex 对象的值。在 main 函数中创建了两个 Complex 对象 a 和 b,并分别进行了加、减、乘、除四种运算,并将结果输出。
阅读全文
相关推荐
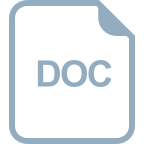
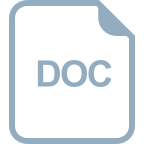
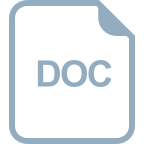
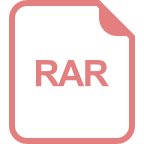
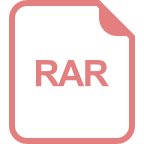
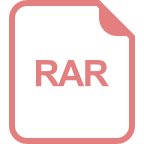
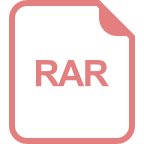
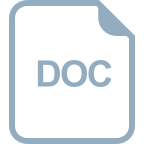
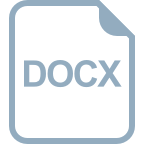
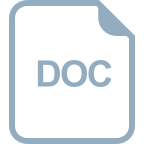
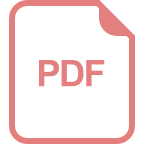
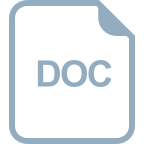
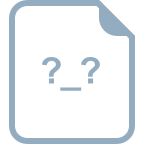
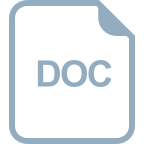
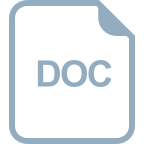


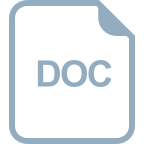