能够实现定点整数的变形补码的加减法的代码
时间: 2024-03-07 09:46:23 浏览: 16
以下是实现定点整数的变形补码的加减法的代码示例(使用C语言):
``` c
#include <stdio.h>
// 定义定点整数位数和小数位数
#define FIXED_POINT_WBITS 16
#define FIXED_POINT_FBITS 8
// 定义定点整数类型
typedef int fixed_t;
// 定义定点整数转换为真值的函数
double fixed_to_double(fixed_t x)
{
return (double)x / (1 << FIXED_POINT_FBITS);
}
// 定义真值转换为定点整数的函数
fixed_t double_to_fixed(double x)
{
return (fixed_t)(x * (1 << FIXED_POINT_FBITS));
}
// 定义定点整数的加法
fixed_t fixed_add(fixed_t x, fixed_t y)
{
fixed_t z = x + y;
// 判断是否溢出,如果溢出,则返回最大或最小值
if ((x > 0 && y > 0 && z < 0) || (x < 0 && y < 0 && z > 0)) {
return (x > 0) ? ((1 << (FIXED_POINT_WBITS - 1)) - 1) : (-1 << (FIXED_POINT_WBITS - 1));
}
return z;
}
// 定义定点整数的减法
fixed_t fixed_sub(fixed_t x, fixed_t y)
{
fixed_t z = x - y;
// 判断是否溢出,如果溢出,则返回最大或最小值
if ((x > 0 && y < 0 && z < 0) || (x < 0 && y > 0 && z > 0)) {
return (x > 0) ? ((1 << (FIXED_POINT_WBITS - 1)) - 1) : (-1 << (FIXED_POINT_WBITS - 1));
}
return z;
}
int main()
{
// 示例:计算 1.5 + 2.25,定点整数位数为16位,小数位数为8位
fixed_t x = double_to_fixed(1.5);
fixed_t y = double_to_fixed(2.25);
fixed_t z = fixed_add(x, y);
printf("1.5 + 2.25 = %f\n", fixed_to_double(z));
// 示例:计算 3.75 - 2.5,定点整数位数为16位,小数位数为8位
x = double_to_fixed(3.75);
y = double_to_fixed(2.5);
z = fixed_sub(x, y);
printf("3.75 - 2.5 = %f\n", fixed_to_double(z));
return 0;
}
```
需要注意的是,以上代码中的定点整数加法和减法均需要进行溢出的判断和处理。另外,定点整数的乘法和除法也需要进行相应的实现。
相关推荐
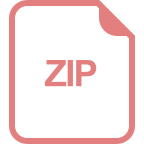
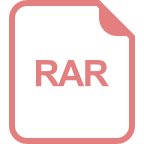














