find_last_of()并举例
时间: 2023-12-16 16:03:01 浏览: 34
`find_last_of()` 是一个字符串操作函数,用于在字符串中查找指定字符集中最后一个出现的字符的位置。它的语法如下:
```
size_t find_last_of (const string& str, size_t pos, const string& chars);
```
其中,`str` 是要查找的字符串,`pos` 是起始查找位置(默认为 `string::npos` 即字符串末尾),`chars` 是要查找的字符集合。
下面是一个示例:
```c++
#include <iostream>
#include <string>
int main() {
std::string str("hello world");
std::size_t pos = str.find_last_of("l");
if (pos != std::string::npos) {
std::cout << "Last 'l' found at position " << pos << std::endl;
}
return 0;
}
```
输出结果为:
```
Last 'l' found at position 9
```
在上面的示例中,`find_last_of()` 函数查找了字符串 `"hello world"` 中最后一个 `'l'` 字符的位置,并返回了该位置的索引值 9。
相关问题
find_last_not_of
`find_last_not_of` 是 C++ 标准库中字符串类 `std::string` 的一个成员函数,用于在字符串中从后往前查找某个字符集合中第一个不在指定位置之前的字符,并返回该字符在字符串中的位置。
函数原型如下:
```cpp
size_t find_last_not_of(const char* s, size_t pos = npos) const;
size_t find_last_not_of(const string& str, size_t pos = npos) const;
size_t find_last_not_of(const char* s, size_t pos, size_t n) const;
size_t find_last_not_of(char c, size_t pos = npos) const;
```
其中 `s` 和 `str` 分别表示要查找的字符集合,`pos` 表示搜索的起始位置,默认为字符串末尾,`n` 表示 `s` 字符数组的长度,`c` 表示要查找的单个字符。如果找到了指定字符集合中的字符,则返回该字符在字符串中的位置;如果找不到,则返回 `string::npos`。
find_first_of和find_last_of及相关函数并举例
除了 `find_first_of`,`std::string` 类中还有一些其他的查找函数,它们的作用都是在字符串中查找指定字符或字符集的位置。下面是几个常用的查找函数及其功能:
1. `find(char ch, size_t pos = 0) const`:在字符串中查找字符 `ch` 的位置,从 `pos` 位置开始查找,默认值为 0。
```cpp
std::string s = "hello, world!";
size_t pos = s.find('o'); // 返回 4
```
2. `rfind(char ch, size_t pos = npos) const`:在字符串中查找字符 `ch` 的位置,从 `pos` 位置往前查找,`npos` 表示从字符串的末尾开始查找。
```cpp
std::string s = "hello, world!";
size_t pos = s.rfind('o'); // 返回 8
```
3. `find_first_of(const char* str, size_t pos = 0) const`:在字符串中查找第一个匹配指定字符集中任意一个字符的位置。
```cpp
std::string s = "hello, world!";
size_t pos = s.find_first_of("ow"); // 返回 4
```
4. `find_last_of(const char* str, size_t pos = npos) const`:在字符串中查找最后一个匹配指定字符集中任意一个字符的位置。
```cpp
std::string s = "hello, world!";
size_t pos = s.find_last_of("ow"); // 返回 9
```
5. `find_first_not_of(const char* str, size_t pos = 0) const`:在字符串中查找第一个不匹配指定字符集中任意一个字符的位置。
```cpp
std::string s = "hello, world!";
size_t pos = s.find_first_not_of("helo, "); // 返回 5
```
6. `find_last_not_of(const char* str, size_t pos = npos) const`:在字符串中查找最后一个不匹配指定字符集中任意一个字符的位置。
```cpp
std::string s = "hello, world!";
size_t pos = s.find_last_not_of("dlrow!"); // 返回 10
```
相关推荐
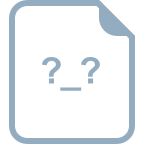
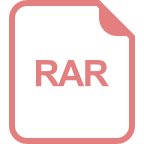
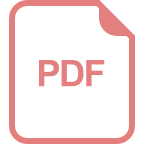












