如何new 一个json对象 c++
时间: 2023-10-28 12:25:38 浏览: 59
在 C 语言中,可以使用以下步骤来创建一个 JSON 对象:
1. 导入必要的头文件:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <json-c/json.h>
```
2. 定义 JSON 对象,并为其分配内存:
```c
json_object *json_obj = json_object_new_object();
```
3. 向 JSON 对象中添加键值对:
```c
json_object_object_add(json_obj, "key1", json_object_new_int(123));
json_object_object_add(json_obj, "key2", json_object_new_string("value"));
// 添加更多的键值对...
```
这里的例子中,添加了两个键值对,一个键为 "key1",对应的值为整数 123,另一个键为 "key2",对应的值为字符串 "value"。你可以根据需要添加更多的键值对。
4. 将 JSON 对象转换为字符串格式:
```c
const char *json_str = json_object_to_json_string(json_obj);
printf("%s\n", json_str);
```
`json_object_to_json_string` 函数将 JSON 对象转换为字符串格式,并将结果存储在 `json_str` 中。你可以通过打印输出来验证生成的 JSON 字符串。
5. 释放内存:
```c
json_object_put(json_obj);
```
在使用完 JSON 对象后,需要调用 `json_object_put` 函数释放分配的内存。
完整示例代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <json-c/json.h>
int main() {
json_object *json_obj = json_object_new_object();
json_object_object_add(json_obj, "key1", json_object_new_int(123));
json_object_object_add(json_obj, "key2", json_object_new_string("value"));
const char *json_str = json_object_to_json_string(json_obj);
printf("%s\n", json_str);
json_object_put(json_obj);
return 0;
}
```
请确保在编译和运行代码时已安装 JSON-C 库。
相关推荐
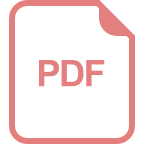
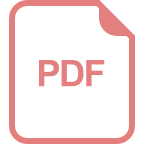
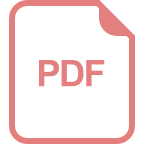














