用Python写一个贪食蛇小游戏
时间: 2023-12-07 12:08:53 浏览: 43
好的,我可以帮你回答这个问题。你可以使用 Python 的 Pygame 模块来编写贪食蛇小游戏。你需要创建一个游戏窗口,初始化贪食蛇和食物的位置,并且编写键盘事件来控制贪食蛇的移动。当贪食蛇吃到食物时,需要更新得分并重新随机生成食物。同时,当贪食蛇碰到边界或自己的身体时,游戏结束。希望这个回答对你有所帮助!
相关问题
用python写一个贪食蛇小游戏
我来给你写一个贪食蛇小游戏:
import pygame
import random
# 定义颜色
red = pygame.Color(255, 0, 0)
green = pygame.Color(0, 255, 0)
black = pygame.Color(0, 0, 0)
white = pygame.Color(255, 255, 255)
# 初始化pygame
pygame.init()
# 创建一个窗口
width = 500
height = 500
screen = pygame.display.set_mode((width, height))
# 设置游戏标题
pygame.display.set_caption("贪食蛇")
# 定义一个贪食蛇类
class Snake:
def __init__(self, color, position):
self.color = color
self.position = position
self.body = []
self.direction = "RIGHT"
# 绘制贪食蛇
def draw_snake(self):
for x, y in self.position:
pygame.draw.rect(screen, self.color, (x, y, 10, 10))
# 更新贪食蛇位置
def update_snake_position(self):
head_x, head_y = self.position[0]
if self.direction == "RIGHT":
new_head_position = (head_x + 10, head_y)
elif self.direction == "LEFT":
new_head_position = (head_x - 10, head_y)
elif self.direction == "UP":
new_head_position = (head_x, head_y - 10)
else:
new_head_position = (head_x, head_y + 10)
self.position.insert(0, new_head_position)
self.position.pop()
# 设置贪食蛇的移动方向
def set_direction(self, direction):
if direction == "RIGHT" and self.direction != "LEFT":
self.direction = direction
elif direction == "LEFT" and self.direction != "RIGHT":
self.direction = direction
elif direction == "UP" and self.direction != "DOWN":
self.direction = direction
elif direction == "DOWN" and self.direction != "UP":
self.direction = direction
# 增加贪食蛇的长度
def add_length(self):
tail_x, tail_y = self.position[-1]
if self.direction == "RIGHT":
self.position.append((tail_x - 10, tail_y))
elif self.direction == "LEFT":
self.position.append((tail_x + 10, tail_y))
elif self.direction == "UP":
self.position.append((tail_x, tail_y + 10))
else:
self.position.append((tail_x, tail_y - 10))
# 定义一个食物类
class Food:
def __init__(self):
self.position = (random.randint(0, 49) * 10, random.randint(0, 49) * 10)
self.is_food_eaten = False
# 绘制食物
def draw_food(self):
pygame.draw.rect(screen, red, (self.position[0], self.position[1], 10, 10))
# 定义游戏的主循环
def game_loop():
# 初始化贪食蛇
snake = Snake(green, [(100, 100), (90, 100), (80, 100)])
# 初始化食物
food = Food()
# 游戏的主循环
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_RIGHT:
snake.set_direction("RIGHT")
elif event.key == pygame.K_LEFT:
snake.set_direction("LEFT")
elif event.key == pygame.K_UP:
snake.set_direction("UP")
elif event.key == pygame.K_DOWN:
snake.set_direction("DOWN")
screen.fill(white)
snake.update_snake_position()
# 判断贪食蛇是否吃到食物
if snake.position[0] == food.position:
food.is_food_eaten = True
snake.add_length()
# 如果食物被吃掉,重新生成食物
if food.is_food_eaten:
food = Food()
# 绘制食物
food.draw_food()
# 绘制贪食蛇
snake.draw_snake()
# 更新画面
pygame.display.update()
game_loop()
请用python写一个贪食蛇小游戏
下面是一个简单的 Python 贪吃蛇小游戏,它使用 Pygame 模块实现。
```python
import pygame
import sys
import random
# Initialize game engine
pygame.init()
# Set up window
WIDTH = 800
HEIGHT = 600
# Colors
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
RED = (255, 0, 0)
# Snake block size
block_size = 10
# FPS
fps = pygame.time.Clock()
# Set up window
window = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("贪吃蛇")
# Game over function
def game_over():
font = pygame.font.SysFont(None, 25)
text = font.render("游戏结束,按 Q 退出游戏", True, RED)
window.blit(text, [WIDTH/2-50, HEIGHT/2])
pygame.display.update()
while True:
for event in pygame.event.get():
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_q:
pygame.quit()
sys.exit()
# Game loop
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# Clear the screen
window.fill(WHITE)
# Draw the snake
# ...
# Draw the food
# ...
# Update screen
pygame.display.update()
# FPS
fps.tick(10)
# Check for collision with food
# ...
# Check for collision with walls or snake body
# ...
# Check if game over
# ...
```
这是一个基础的游戏框架,您可以根据自己的需求扩展它。例如,您可以使用键盘事件处理玩家的控制,并在每帧中更新蛇的位置。
希望对您有所帮助!
相关推荐







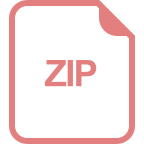
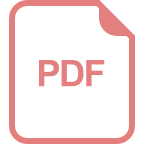
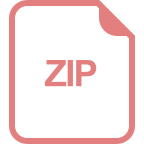
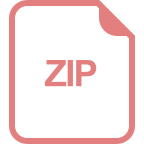