帮我用python写一个贪食蛇代码
时间: 2024-01-03 19:04:34 浏览: 76
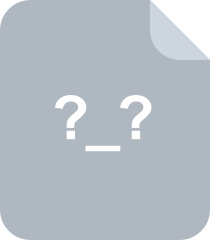
贪吃蛇python代码
好的,以下是 Python 实现的贪吃蛇游戏代码,供您参考:
```Python
import pygame
import random
pygame.init()
# 设置游戏窗口大小
WIDTH, HEIGHT = 480, 480
WINDOW = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("贪吃蛇")
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
# 定义贪吃蛇类
class Snake:
def __init__(self):
self.squares = [(220, 200), (210, 200), (200, 200)]
self.direction = "RIGHT"
self.length = 3
def move(self):
x, y = self.squares[0]
if self.direction == "UP":
y -= 10
elif self.direction == "DOWN":
y += 10
elif self.direction == "LEFT":
x -= 10
elif self.direction == "RIGHT":
x += 10
self.squares.insert(0, (x, y))
if len(self.squares) > self.length:
self.squares.pop()
def draw(self):
for square in self.squares:
pygame.draw.rect(WINDOW, GREEN, (square[0], square[1], 10, 10))
def turn(self, direction):
if direction == "UP" and self.direction != "DOWN":
self.direction = "UP"
elif direction == "DOWN" and self.direction != "UP":
self.direction = "DOWN"
elif direction == "LEFT" and self.direction != "RIGHT":
self.direction = "LEFT"
elif direction == "RIGHT" and self.direction != "LEFT":
self.direction = "RIGHT"
def eat(self):
self.length += 1
def reset(self):
self.squares = [(220, 200), (210, 200), (200, 200)]
self.direction = "RIGHT"
self.length = 3
# 定义食物类
class Food:
def __init__(self):
self.x, self.y = random.randrange(0, WIDTH-10, 10), random.randrange(0, HEIGHT-10, 10)
self.color = RED
def draw(self):
pygame.draw.rect(WINDOW, self.color, (self.x, self.y, 10, 10))
def respawn(self):
self.x, self.y = random.randrange(0, WIDTH-10, 10), random.randrange(0, HEIGHT-10, 10)
# 定义游戏循环
def gameLoop():
running = True
clock = pygame.time.Clock()
snake = Snake()
food = Food()
score = 0
while running:
clock.tick(20)
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
snake.turn("UP")
elif event.key == pygame.K_DOWN:
snake.turn("DOWN")
elif event.key == pygame.K_LEFT:
snake.turn("LEFT")
elif event.key == pygame.K_RIGHT:
snake.turn("RIGHT")
snake.move()
# 贪吃蛇与食物碰撞检测
if snake.squares[0][0] == food.x and snake.squares[0][1] == food.y:
food.respawn()
snake.eat()
score += 10
# 贪吃蛇与墙壁碰撞检测
if snake.squares[0][0] < 0 or snake.squares[0][0] > WIDTH-10 or snake.squares[0][1] < 0 or snake.squares[0][1] > HEIGHT-10:
score = 0
snake.reset()
# 贪吃蛇与自身碰撞检测
for square in snake.squares[1:]:
if snake.squares[0][0] == square[0] and snake.squares[0][1] == square[1]:
score = 0
snake.reset()
# 重绘游戏窗口
WINDOW.fill(BLACK)
snake.draw()
food.draw()
# 显示得分
font = pygame.font.SysFont(None, 24)
text = font.render("Score: " + str(score), True, WHITE)
WINDOW.blit(text, (10, 10))
pygame.display.update()
pygame.quit()
quit()
gameLoop()
```
使用方法:
1. 打开 Python 环境,在命令行中运行上述代码。
2. 运行后即可开始游戏,使用方向键控制贪吃蛇移动。
3. 如果贪吃蛇与食物碰撞,得分加 10 分,食物会重新生成;如果贪吃蛇与墙壁或自身碰撞,得分清零,游戏重新开始。
希望您能顺利运行此代码,如有问题,欢迎向我提问。
阅读全文
相关推荐

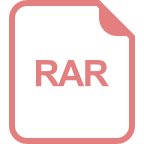










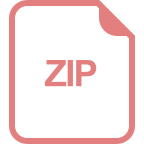
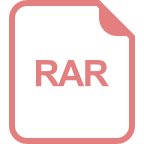