用python写贪食蛇源代码
时间: 2024-01-03 15:02:12 浏览: 38
贪食蛇(Snake)是一种经典的游戏,玩家通过控制蛇的移动来吃食物,蛇的长度会不断增长。下面是一个由Python编写的贪食蛇源代码示例:
```python
import pygame
import random
WIDTH, HEIGHT = 640, 480
GRID_SIZE = 20
GRID_WIDTH = WIDTH // GRID_SIZE
GRID_HEIGHT = HEIGHT // GRID_SIZE
UP = (0, -1)
DOWN = (0, 1)
LEFT = (-1, 0)
RIGHT = (1, 0)
def draw_snake(snake):
for block in snake:
rect = pygame.Rect(block[0] * GRID_SIZE, block[1] * GRID_SIZE, GRID_SIZE, GRID_SIZE)
pygame.draw.rect(screen, (0, 255, 0), rect)
def move_snake(snake, direction):
head = (snake[0][0] + direction[0], snake[0][1] + direction[1])
snake.insert(0, head)
snake.pop()
def generate_food():
while True:
x = random.randint(0, GRID_WIDTH - 1)
y = random.randint(0, GRID_HEIGHT - 1)
if (x, y) not in snake:
return (x, y)
pygame.init()
screen = pygame.display.set_mode((WIDTH, HEIGHT))
clock = pygame.time.Clock()
snake = [(GRID_WIDTH // 2, GRID_HEIGHT // 2)]
direction = RIGHT
food = generate_food()
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_w and direction != DOWN:
direction = UP
elif event.key == pygame.K_s and direction != UP:
direction = DOWN
elif event.key == pygame.K_a and direction != RIGHT:
direction = LEFT
elif event.key == pygame.K_d and direction != LEFT:
direction = RIGHT
move_snake(snake, direction)
if snake[0] == food:
food = generate_food()
else:
snake.pop()
screen.fill((0, 0, 0))
draw_snake(snake)
pygame.draw.rect(screen, (255, 0, 0), pygame.Rect(food[0] * GRID_SIZE, food[1] * GRID_SIZE, GRID_SIZE, GRID_SIZE))
pygame.display.flip()
clock.tick(10)
pygame.quit()
```
这段代码使用了pygame库来实现游戏的绘图与事件处理部分,snake列表存储贪食蛇的身体块的坐标,direction表示蛇的移动方向。通过键盘事件来控制蛇的移动方向,每帧更新蛇的位置并绘制在屏幕上,同时检测蛇是否吃到食物并更新食物的位置。游戏结束后调用`pygame.quit()`来退出pygame。
相关推荐
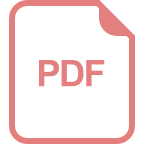
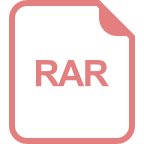














