使用C语言将灰度图像自适应直方图均衡化的方法来提升对比度,要求不使用opencv库
时间: 2023-12-12 16:05:14 浏览: 35
灰度图像的自适应直方图均衡化可以通过以下步骤实现:
1. 读取灰度图像并将其存储为一维数组;
2. 定义一个滑动窗口,以每个像素点为中心,计算该窗口内像素的直方图;
3. 计算该窗口内像素的累积分布函数,并将其映射到0到255的范围内;
4. 将窗口中心像素点的像素值替换为其映射后的值,然后将滑动窗口移动到下一个像素点;
5. 将处理后的像素值存储为一维数组,并将其转换为图像格式并保存。
下面是一个示例代码,用于实现灰度图像的自适应直方图均衡化:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define IMAGE_WIDTH 512
#define IMAGE_HEIGHT 512
#define WINDOW_SIZE 15
#define HISTOGRAM_SIZE 256
// 读取灰度图像
void read_image(const char *filename, unsigned char *image) {
FILE *fp;
if ((fp = fopen(filename, "rb")) == NULL) {
printf("Error: image cannot be opened!\n");
exit(1);
}
fread(image, sizeof(unsigned char), IMAGE_WIDTH * IMAGE_HEIGHT, fp);
fclose(fp);
}
// 写入灰度图像
void write_image(const char *filename, unsigned char *image) {
FILE *fp;
if ((fp = fopen(filename, "wb")) == NULL) {
printf("Error: cannot write image!\n");
exit(1);
}
fwrite(image, sizeof(unsigned char), IMAGE_WIDTH * IMAGE_HEIGHT, fp);
fclose(fp);
}
// 计算像素值的直方图
void calculate_histogram(unsigned char *image, int *histogram, int x, int y) {
int i, j;
for (i = 0; i < HISTOGRAM_SIZE; i++) {
histogram[i] = 0;
}
for (i = x - WINDOW_SIZE / 2; i <= x + WINDOW_SIZE / 2; i++) {
for (j = y - WINDOW_SIZE / 2; j <= y + WINDOW_SIZE / 2; j++) {
if (i >= 0 && i < IMAGE_HEIGHT && j >= 0 && j < IMAGE_WIDTH) {
histogram[image[i * IMAGE_WIDTH + j]]++;
}
}
}
}
// 计算直方图的累积分布函数
void calculate_cdf(int *histogram, float *cdf) {
int i, sum = 0;
for (i = 0; i < HISTOGRAM_SIZE; i++) {
sum += histogram[i];
cdf[i] = (float)sum / (WINDOW_SIZE * WINDOW_SIZE);
}
}
// 映射像素值到0到255的范围内
unsigned char map_pixel_value(unsigned char pixel, float *cdf) {
return (unsigned char)(cdf[pixel] * 255.0);
}
// 自适应直方图均衡化
void adaptive_histogram_equalization(unsigned char *image, unsigned char *result) {
int x, y, i;
int histogram[HISTOGRAM_SIZE];
float cdf[HISTOGRAM_SIZE];
for (x = 0; x < IMAGE_HEIGHT; x++) {
for (y = 0; y < IMAGE_WIDTH; y++) {
calculate_histogram(image, histogram, x, y);
calculate_cdf(histogram, cdf);
result[x * IMAGE_WIDTH + y] = map_pixel_value(image[x * IMAGE_WIDTH + y], cdf);
}
}
}
int main() {
unsigned char image[IMAGE_WIDTH * IMAGE_HEIGHT];
unsigned char result[IMAGE_WIDTH * IMAGE_HEIGHT];
read_image("input.raw", image);
adaptive_histogram_equalization(image, result);
write_image("output.raw", result);
return 0;
}
```
注意:在实际应用中,自适应直方图均衡化的窗口大小应该根据实际情况进行调整,以达到最佳的效果。
相关推荐
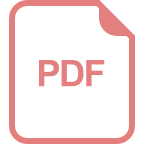
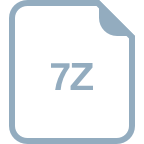
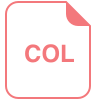
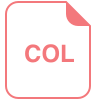
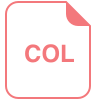
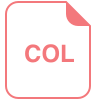
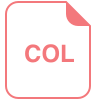









