Python剪刀石头布的GUI精美开发
时间: 2023-08-27 07:35:23 浏览: 93
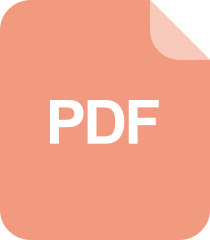
python实现石头剪刀布程序
如果你想要开发一个更加精美的剪刀石头布游戏,可以使用Python的GUI库Pygame来实现。以下是一个简单的示例代码:
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 定义颜色
white = (255, 255, 255)
black = (0, 0, 0)
red = (255, 0, 0)
# 定义窗口大小
window_size = (800, 600)
# 创建窗口
window = pygame.display.set_mode(window_size)
pygame.display.set_caption("剪刀石头布")
# 加载图片
image_scissors = pygame.image.load("scissors.png")
image_rock = pygame.image.load("rock.png")
image_paper = pygame.image.load("paper.png")
# 缩放图片
image_scissors = pygame.transform.scale(image_scissors, (200, 200))
image_rock = pygame.transform.scale(image_rock, (200, 200))
image_paper = pygame.transform.scale(image_paper, (200, 200))
# 定义字体
font = pygame.font.Font(None, 50)
# 定义函数,判断胜负
def check_win(player_choice, computer_choice):
if player_choice == computer_choice:
return "平局"
elif (player_choice == "剪刀" and computer_choice == "石头") or \
(player_choice == "石头" and computer_choice == "布") or \
(player_choice == "布" and computer_choice == "剪刀"):
return "你输了"
else:
return "你赢了"
# 定义函数,生成电脑出拳
def generate_computer_choice():
choices = ["剪刀", "石头", "布"]
return random.choice(choices)
# 创建标签和按钮
text_instruction = font.render("请选择:", True, black)
button_scissors = image_scissors.get_rect(center=(200, 400))
button_rock = image_rock.get_rect(center=(400, 400))
button_paper = image_paper.get_rect(center=(600, 400))
# 进入游戏循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.MOUSEBUTTONDOWN:
mouse_pos = pygame.mouse.get_pos()
if button_scissors.collidepoint(mouse_pos):
player_choice = "剪刀"
elif button_rock.collidepoint(mouse_pos):
player_choice = "石头"
elif button_paper.collidepoint(mouse_pos):
player_choice = "布"
else:
player_choice = None
# 生成电脑出拳
computer_choice = generate_computer_choice()
# 判断胜负
if player_choice is None:
text_result = font.render("", True, red)
else:
result = check_win(player_choice, computer_choice)
text_result = font.render("电脑出了%s,%s" % (computer_choice, result), True, red)
# 绘制界面
window.fill(white)
window.blit(text_instruction, (280, 100))
window.blit(image_scissors, button_scissors)
window.blit(image_rock, button_rock)
window.blit(image_paper, button_paper)
window.blit(text_result, (200, 300))
pygame.display.update()
# 退出Pygame
pygame.quit()
```
这段代码会创建一个更加精美的窗口,其中包括三个按钮和一个标签。玩家可以点击按钮选择出拳,然后电脑随机出拳,计算胜负并显示结果。你可以根据自己的需求进行修改和扩展。注意,这段代码需要准备剪刀、石头、布三张图片,并将它们命名为scissors.png、rock.png和paper.png,放在同一个目录下。
阅读全文
相关推荐
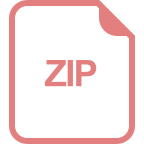
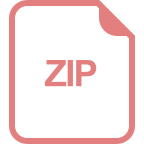
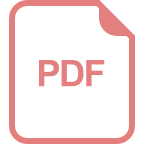
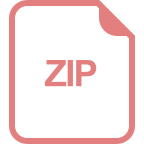
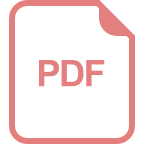
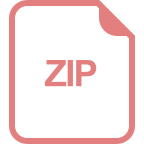
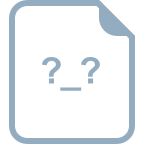
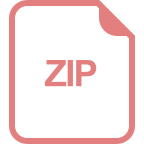
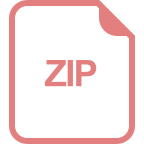
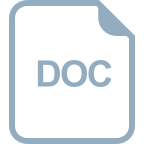
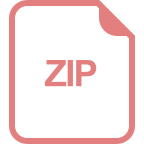
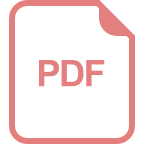
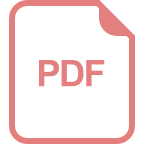
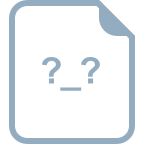