构建贝叶斯信念网络模型对鸢尾花数据进行分类,对模型的准确率进行分析
时间: 2024-01-09 07:19:38 浏览: 75
首先,让我们导入需要的库和数据集:
```python
import numpy as np
from pomegranate import BayesianNetwork
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
# load iris dataset
iris = load_iris()
X, y = iris.data, iris.target
```
接下来,我们将数据集分成训练集和测试集:
```python
# split data into train and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
然后,我们可以构建贝叶斯信念网络模型。在这个例子中,我们使用 `pomegranate` 库中的 `BayesianNetwork` 类来构建模型。我们将使用 `fit` 方法来拟合训练数据:
```python
# create Bayesian Network model
model = BayesianNetwork.from_samples(X_train, algorithm='exact')
```
在训练模型之后,我们可以使用 `predict` 方法来对测试数据进行预测:
```python
# predict on test data
y_pred = np.argmax([model.predict(X_test[i, :])[0] for i in range(X_test.shape[0])], axis=1)
```
最后,我们可以计算模型的准确率:
```python
# calculate accuracy
accuracy = np.sum(y_pred == y_test) / y_test.shape[0]
print(f"Accuracy: {accuracy:.2f}")
```
完整的代码如下:
```python
import numpy as np
from pomegranate import BayesianNetwork
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
# load iris dataset
iris = load_iris()
X, y = iris.data, iris.target
# split data into train and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# create Bayesian Network model
model = BayesianNetwork.from_samples(X_train, algorithm='exact')
# predict on test data
y_pred = np.argmax([model.predict(X_test[i, :])[0] for i in range(X_test.shape[0])], axis=1)
# calculate accuracy
accuracy = np.sum(y_pred == y_test) / y_test.shape[0]
print(f"Accuracy: {accuracy:.2f}")
```
你可以运行这段代码,得到模型的准确率。
阅读全文
相关推荐
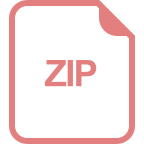





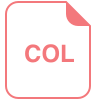
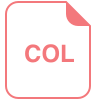
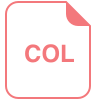

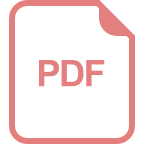
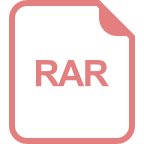