请给出相应的具体代码
时间: 2024-10-12 12:06:58 浏览: 23
在处理无人机实时位置信息的代码示例中,我们通常会创建一个无人机类(Drone),包含状态(location, current_task)和行为(assign_task, update_location)。这里是一个简单的Python伪代码示例,假设我们使用优先队列来存储任务,并且使用Dijkstra's算法作为近似A*搜索的简化版本:
```python
import heapq
class Drone:
def __init__(self, id, initial_location):
self.id = id
self.location = initial_location
self.task_queue = PriorityQueue()
def assign_task(self, task, graph):
self.current_task = task
self.location = task.start_location # 假设任务开始于起点
heapq.heappush(self.task_queue, (0, task.end_location)) # 将目标地点加入优先队列,0表示初始距离
def update_location(self, new_location):
self.location = new_location
# 更新任务队列的距离估计,如果有必要,可以基于地图数据重新评估路径
_, next_node = heapq.heappop(self.task_queue) # 取出当前最短路径节点
cost_to_next = calculate_cost(new_location, next_node)
heapq.heappush(self.task_queue, (cost_to_next, next_node))
# 使用Dijkstra's算法简化版
class PriorityQueue:
# ...省略具体实现...
def dijkstra(graph, start):
distances = {node: float('infinity') for node in graph}
distances[start] = 0
queue = [(0, start)]
while queue:
curr_dist, curr_node = heapq.heappop(queue)
if curr_dist > distances[curr_node]:
continue # 已经找到更优路径,跳过
for neighbor, weight in graph[curr_node].items():
distance = curr_dist + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(queue, (distance, neighbor))
return distances
# 使用示例:
graph = construct_graph(map_data) # 构建无人机活动区域的地图图谱
drone1 = Drone(1, initial_location)
for task in tasks:
drone1.assign_task(task, graph)
# 调用update_location新获得的位置信息
```
请注意,这只是一个基础框架,实际应用中可能需要添加更多的错误检查和复杂逻辑,例如处理多路任务、实时通信限制等。
阅读全文
相关推荐
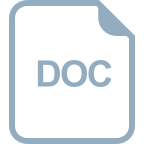
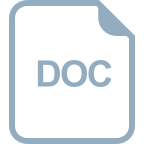
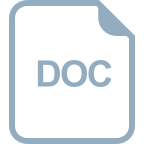
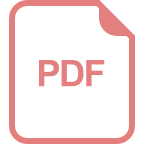
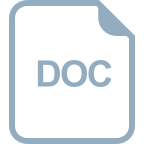
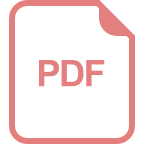
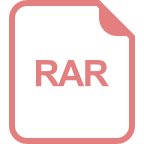
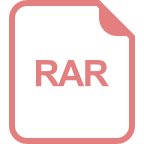
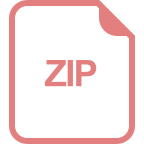
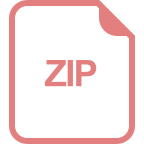
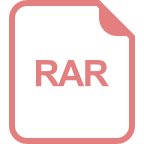
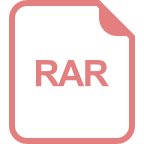
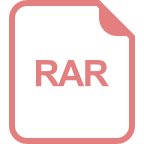
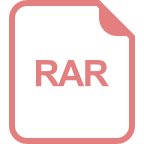
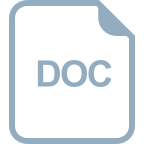
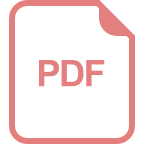
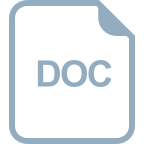
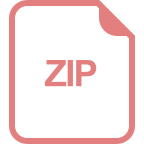
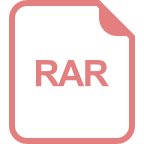