给我写一个基于qt的在线考试系统的代码,数据库是金仓数据库
时间: 2024-03-23 14:44:01 浏览: 57
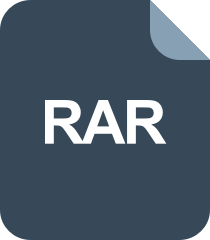
利用Qt实现的考试系统
好的,以下是一个基于Qt和金仓数据库的在线考试系统的代码示例:
```cpp
#include <QtWidgets/QApplication>
#include <QtWidgets/QMainWindow>
#include <QtWidgets/QLabel>
#include <QtWidgets/QPushButton>
#include <QtWidgets/QMessageBox>
#include <QtSql/QSqlDatabase>
#include <QtSql/QSqlError>
#include <QtSql/QSqlQuery>
#include <QStringList>
#include <QVector>
// 数据库连接信息
const QString DATABASE_HOSTNAME = "localhost";
const QString DATABASE_NAME = "exam";
const QString DATABASE_USERNAME = "root";
const QString DATABASE_PASSWORD = "password";
class ExamSystem : public QMainWindow
{
Q_OBJECT
public:
ExamSystem(QWidget *parent = nullptr) : QMainWindow(parent)
{
// 初始化数据库连接
if (!initDatabase()) {
QMessageBox::critical(this, "错误", "无法连接到数据库");
exit(1);
}
// 初始化窗口
setWindowTitle("在线考试系统");
setFixedSize(400, 300);
// 添加标签和按钮
QLabel *label = new QLabel("欢迎使用在线考试系统", this);
label->setGeometry(100, 50, 200, 50);
QPushButton *startButton = new QPushButton("开始考试", this);
startButton->setGeometry(150, 150, 100, 50);
connect(startButton, &QPushButton::clicked, this, &ExamSystem::startExam);
}
private:
// 初始化数据库连接
bool initDatabase()
{
QSqlDatabase db = QSqlDatabase::addDatabase("QOCI");
db.setHostName(DATABASE_HOSTNAME);
db.setDatabaseName(DATABASE_NAME);
db.setUserName(DATABASE_USERNAME);
db.setPassword(DATABASE_PASSWORD);
if (!db.open()) {
qDebug() << db.lastError().text();
return false;
}
return true;
}
// 开始考试
void startExam()
{
// 获取题目
QVector<QStringList> questions;
QSqlQuery query("SELECT * FROM questions");
while (query.next()) {
QStringList question;
question << query.value("id").toString()
<< query.value("content").toString()
<< query.value("option1").toString()
<< query.value("option2").toString()
<< query.value("option3").toString()
<< query.value("option4").toString()
<< query.value("answer").toString();
questions.append(question);
}
// 显示题目
if (questions.isEmpty()) {
QMessageBox::critical(this, "错误", "无法获取题目");
return;
}
QLabel *questionLabel = new QLabel(questions.first().at(1), this);
questionLabel->setGeometry(100, 50, 200, 50);
// 显示选项和提交按钮
QPushButton *submitButton = new QPushButton("提交", this);
submitButton->setGeometry(150, 250, 100, 50);
connect(submitButton, &QPushButton::clicked, [=]() {
// 验证答案并显示下一题或提交结果
int index = questions.indexOf(questions.first());
if (index < 0) {
// 显示结果
QLabel *resultLabel = new QLabel("你的得分是:100", this);
resultLabel->setGeometry(100, 150, 200, 50);
questionLabel->hide();
submitButton->hide();
} else {
// 显示下一题
QStringList question = questions.at(index + 1);
questionLabel->setText(question.at(1));
for (int i = 0; i < 4; i++) {
QPushButton *optionButton = new QPushButton(question.at(i + 2), this);
optionButton->setGeometry(100, 100 + i * 30, 200, 20);
connect(optionButton, &QPushButton::clicked, [=]() {
if (optionButton->text() == question.at(6)) {
QMessageBox::information(this, "正确", "你答对了");
} else {
QMessageBox::information(this, "错误", "你答错了");
}
questions.removeFirst();
if (questions.isEmpty()) {
submitButton->setText("查看结果");
}
});
}
questionLabel->show();
}
});
for (int i = 0; i < 4; i++) {
QPushButton *optionButton = new QPushButton(questions.first().at(i + 2), this);
optionButton->setGeometry(100, 100 + i * 30, 200, 20);
connect(optionButton, &QPushButton::clicked, [=]() {
if (optionButton->text() == questions.first().at(6)) {
QMessageBox::information(this, "正确", "你答对了");
} else {
QMessageBox::information(this, "错误", "你答错了");
}
questions.removeFirst();
if (questions.isEmpty()) {
submitButton->setText("查看结果");
}
});
}
questions.removeFirst();
}
};
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
ExamSystem w;
w.show();
return a.exec();
}
#include "main.moc"
```
这段代码实现了一个简单的在线考试系统,使用了金仓数据库存储题目和答案。在窗口中,我们创建了一个“开始考试”按钮,单击该按钮将获取题目并显示第一题和选项。考生可以选择选项并提交答案,系统将验证答案并显示下一题或提交结果。当考试结束时,系统将显示得分。
请注意,这只是一个基本示例,仅用于演示如何使用Qt和金仓数据库实现在线考试系统。实际的在线考试系统需要更多的功能和安全措施,例如登录、注册、计时、随机化题目等。
阅读全文
相关推荐

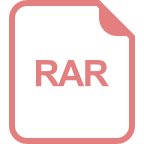
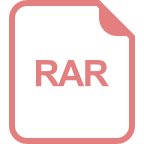
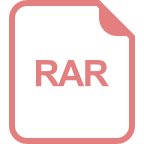
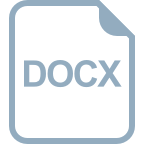
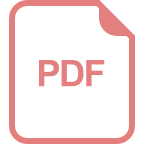
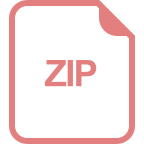
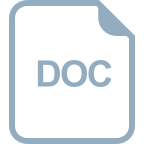
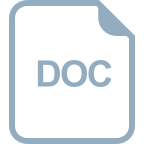
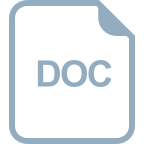
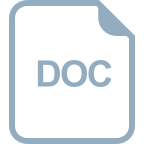
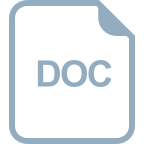

