好,其中 date 是归还日期 格式是 年-月-日 ,给我写一个函数,即将到期的图书,还要有一个参数临期几天的。使用C语言写
时间: 2024-04-29 13:26:47 浏览: 117
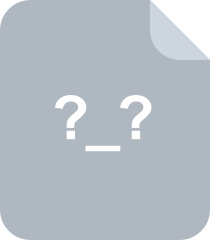
年月日c语言
以下是一个示例函数:
```
#include <stdio.h>
#include <time.h>
typedef struct {
char name[50];
char author[50];
char publisher[50];
char ISBN[20];
char category[20];
char borrower[50];
char borrow_date[11];
char return_date[11];
} BOOK;
int days_until_due(char *return_date, int warning_days) {
struct tm due_time = {0};
time_t now, due;
sscanf(return_date, "%d-%d-%d", &due_time.tm_year, &due_time.tm_mon, &due_time.tm_mday);
due_time.tm_year -= 1900;
due_time.tm_mon--;
time(&now);
due = mktime(&due_time);
return (int) (difftime(due, now) / (24 * 3600)) - warning_days;
}
void print_books_near_due(BOOK *books, int num_books, int warning_days) {
int i, days_left;
printf("The following books will be due soon:\n");
for (i = 0; i < num_books; i++) {
if (books[i].borrower[0] == '\0') continue;
days_left = days_until_due(books[i].return_date, warning_days);
if (days_left <= 0)
printf("%s, borrowed by %s, is due today.\n", books[i].name, books[i].borrower);
else if (days_left <= warning_days)
printf("%s, borrowed by %s, will be due in %d day(s).\n", books[i].name, books[i].borrower, days_left);
}
}
int main() {
BOOK library[] = {
{"Introduction to C Programming", "John Smith", "Publisher A", "ISBN123", "Programming", "Alice", "2022-01-01", "2022-02-01"},
{"Learning Python", "Jane Doe", "Publisher B", "ISBN345", "Programming", "Bob", "2022-01-03", "2022-02-03"},
{"Pride and Prejudice", "Jane Austen", "Publisher C", "ISBN678", "Fiction", "Carol", "2022-01-05", "2022-02-05"},
{"The History of China", "David Lee", "Publisher D", "ISBN910", "History", "", "2022-01-07", "2022-02-07"}
};
int num_books = sizeof(library) / sizeof(BOOK);
int warning_days = 5;
print_books_near_due(library, num_books, warning_days);
return 0;
}
```
该函数依赖于 `days_until_due` 函数,该函数接受一个日期字符串和一个警告天数,并返回收到警告时距离到期的天数。主函数中的 `print_books_near_due` 函数检查每个书籍的归还日期,并打印将到期或已到期的书籍。在此函数中,我们忽略未被借阅的书籍。
阅读全文
相关推荐
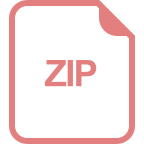
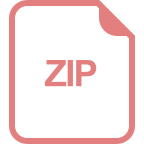
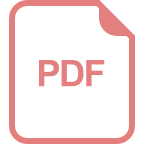
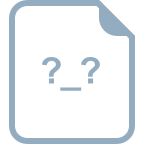
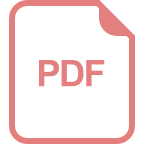
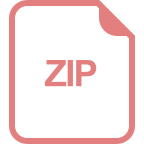
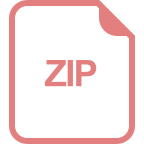
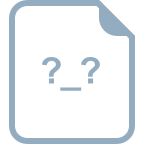
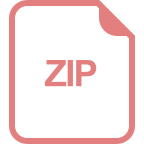
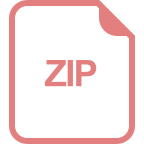
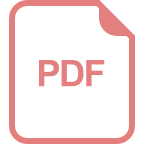
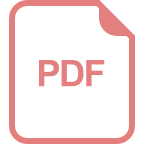
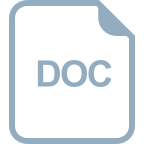
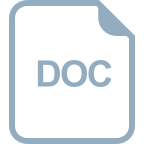
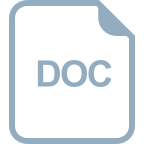