回调函数实现滤波算法代码
时间: 2024-10-30 10:02:15 浏览: 2
回调函数是一种常见的编程技术,常用于异步操作中处理结果。在滤波算法中,尤其是信号处理或数据流应用中,我们可以创建一个函数,将其作为参数传递给另一个函数,当满足某些条件(比如数据达到预设阈值或完成计算)时,这个函数就会被调用。下面是一个简单的JavaScript示例,展示如何使用回调函数实现低通滤波:
```javascript
function applyLowPassFilter(data, callback) {
// 模拟低通滤波过程...
let filteredData = filter(data, { cutoffFrequency: 10 }); // 这里只是一个模拟,实际需要依据具体的滤波库
setTimeout(() => {
// 当滤波完成后,调用回调函数并传入过滤后的数据
callback(filteredData);
}, 100); // 假设滤波过程需要100毫秒
}
// 使用回调函数的示例
const originalData = [1, 2, 3, 4, 5]; // 假设这是输入的数据
applyLowPassFilter(originalData, (filteredOutput) => {
console.log('Filtered output:', filteredOutput);
//
相关问题
生成一段基于ROS的扩展卡尔曼滤波算法代码,要求订阅小车的里程计数据并通过扩展卡尔曼滤波算法来估算出下一时刻小车的x轴和y轴坐标
以下是一个基于ROS的扩展卡尔曼滤波算法示例代码,用于订阅小车的里程计数据并通过扩展卡尔曼滤波算法来估算下一时刻小车的x轴和y轴坐标。
```cpp
#include <ros/ros.h>
#include <nav_msgs/Odometry.h>
#include <geometry_msgs/PointStamped.h>
#include <geometry_msgs/PoseWithCovarianceStamped.h>
#include <tf/transform_datatypes.h>
#include <Eigen/Core>
#include <Eigen/LU>
// Define the state vector and covariance matrix
Eigen::VectorXd x(3);
Eigen::MatrixXd P(3, 3);
// Define the measurement noise covariance matrix R
Eigen::MatrixXd R(2, 2);
// Define the process noise covariance matrix Q
Eigen::MatrixXd Q(3, 3);
// Define the state transition matrix F and measurement matrix H
Eigen::MatrixXd F(3, 3);
Eigen::MatrixXd H(2, 3);
// Initialize the flag for the first time receiving odom data
bool odom_init = false;
// Initialize the last time stamp of the odom data
ros::Time last_odom_time;
// Callback function for the odom data
void odomCallback(const nav_msgs::Odometry::ConstPtr& odom_msg)
{
// Initialize the state vector x and covariance matrix P using the first odom data
if (!odom_init)
{
x << odom_msg->pose.pose.position.x,
odom_msg->pose.pose.position.y,
tf::getYaw(odom_msg->pose.pose.orientation);
P.setIdentity();
odom_init = true;
}
// Compute the time interval between the current and last odom data
double delta_t = (odom_msg->header.stamp - last_odom_time).toSec();
last_odom_time = odom_msg->header.stamp;
// Update the state transition matrix F and process noise covariance matrix Q
F << 1, 0, -sin(x(2))*odom_msg->twist.twist.linear.x*delta_t,
0, 1, cos(x(2))*odom_msg->twist.twist.linear.x*delta_t,
0, 0, 1;
Q << pow(delta_t, 4)/4, 0, pow(delta_t, 3)/2,
0, pow(delta_t, 4)/4, pow(delta_t, 3)/2,
pow(delta_t, 3)/2, pow(delta_t, 3)/2, pow(delta_t, 2);
// Predict the state vector x and covariance matrix P
Eigen::VectorXd x_pred = F*x;
Eigen::MatrixXd P_pred = F*P*F.transpose() + Q;
// Update the measurement matrix H and measurement noise covariance matrix R
H << 1, 0, 0,
0, 1, 0;
R << 0.1, 0,
0, 0.1;
// Convert the odom data to a PointStamped message for publishing
geometry_msgs::PointStamped point_msg;
point_msg.header.stamp = odom_msg->header.stamp;
point_msg.header.frame_id = odom_msg->header.frame_id;
point_msg.point.x = x_pred(0);
point_msg.point.y = x_pred(1);
point_msg.point.z = 0;
// Convert the state vector x and covariance matrix P to a PoseWithCovarianceStamped message for publishing
geometry_msgs::PoseWithCovarianceStamped pose_msg;
pose_msg.header.stamp = odom_msg->header.stamp;
pose_msg.header.frame_id = odom_msg->header.frame_id;
pose_msg.pose.pose.position.x = x_pred(0);
pose_msg.pose.pose.position.y = x_pred(1);
pose_msg.pose.pose.position.z = 0;
pose_msg.pose.pose.orientation = tf::createQuaternionMsgFromYaw(x_pred(2));
pose_msg.pose.covariance[0] = P_pred(0, 0);
pose_msg.pose.covariance[1] = P_pred(0, 1);
pose_msg.pose.covariance[5] = P_pred(1, 1);
pose_msg.pose.covariance[6] = P_pred(0, 2);
pose_msg.pose.covariance[7] = P_pred(1, 2);
pose_msg.pose.covariance[11] = P_pred(2, 2);
// Publish the PointStamped and PoseWithCovarianceStamped messages
ros::NodeHandle nh;
ros::Publisher point_pub = nh.advertise<geometry_msgs::PointStamped>("pose_estimate", 1);
ros::Publisher pose_pub = nh.advertise<geometry_msgs::PoseWithCovarianceStamped>("pose_estimate_cov", 1);
point_pub.publish(point_msg);
pose_pub.publish(pose_msg);
// Update the state vector x and covariance matrix P using the measurement data
Eigen::VectorXd z(2);
z << odom_msg->pose.pose.position.x,
odom_msg->pose.pose.position.y;
Eigen::VectorXd y = z - H*x_pred;
Eigen::MatrixXd S = H*P_pred*H.transpose() + R;
Eigen::MatrixXd K = P_pred*H.transpose()*S.inverse();
x = x_pred + K*y;
P = (Eigen::MatrixXd::Identity(3, 3) - K*H)*P_pred;
}
int main(int argc, char** argv)
{
ros::init(argc, argv, "ekf_node");
ros::NodeHandle nh;
// Subscribe to the odom data
ros::Subscriber odom_sub = nh.subscribe("odom", 1, odomCallback);
ros::spin();
return 0;
}
```
在这个示例代码中,我们使用了Eigen库来实现矩阵运算。首先,我们定义了状态向量x、协方差矩阵P、测量噪声协方差矩阵R和过程噪声协方差矩阵Q。然后,在odomCallback回调函数中,我们根据接收到的里程计数据更新状态向量x和协方差矩阵P。接着,我们根据更新后的状态向量x和协方差矩阵P,用扩展卡尔曼滤波算法预测下一时刻小车的x轴和y轴坐标,并将预测结果发布为一个PointStamped和PoseWithCovarianceStamped消息。最后,我们使用接收到的里程计数据来更新状态向量x和协方差矩阵P。
这个示例代码中假设小车只能直线行驶,因此状态向量x只包含了小车的x轴和y轴坐标以及方向角度。如果需要考虑小车的转向和加速度等因素,需要根据实际情况修改状态向量、协方差矩阵、过程噪声协方差矩阵和状态转移矩阵。
STM32G071CBT6智能小车 要求实现循迹 避障 代码
STM32G071CBT6是一款基于STM32G0微控制器系列的单片机,通常用于嵌入式系统,如智能小车项目。要实现循迹和避障功能,你需要完成以下几个步骤:
1. **硬件准备**:
- 安装传感器:比如红外循迹传感器和超声波雷达模块,用于检测轨道和障碍物。
- 连接电机驱动:通过PWM信号控制步进电机或DC电机。
2. **软件库配置**:
- 设置STM32G0的GPIO、定时器和中断处理程序。
- 使用HAL库或FreeRTOS等RTOS框架,简化I/O操作和任务管理。
3. **循迹算法**:
- 对红外传感器的数据进行处理,计算出当前行驶方向。你可以使用卡尔曼滤波或者其他滤波技术提高精度。
- 编写PID(比例积分微分)控制器来控制车辆沿着传感器读到的轨迹线行驶。
4. **避障算法**:
- 当超声波雷达检测到前方有障碍物时,计算距离并决定是否需要停止、改变方向或绕过。
- 可能会采用模糊逻辑或机器学习算法对障碍物进行识别和决策。
5. **主循环和任务管理**:
- 主程序中不断读取传感器数据,根据实时情况更新循迹和避障策略。
- 结合中断服务程序,实现实时响应。
6. **代码示例**:
- 由于这是一个复杂的项目,具体的代码片段可能包含初始化设备、数据处理函数、PID控制函数以及障碍物检测回调等部分。这里给出的部分伪代码示例:
```cpp
// 初始化传感器和电机
void initSensorsAndMotors();
// 循迹算法
void trackLine();
// 避障算法
void avoidObstacle();
int main() {
initSensorsAndMotors();
while (true) {
if (isTracking()) {
trackLine();
} else if (detectObstacle()) {
avoidObstacle();
}
// 更新电机驱动
driveMotors();
}
}
// 中断处理函数
void ultrasonicSensorInterrupt() {
// 检测障碍物并更新避障状态
}
```
阅读全文
相关推荐
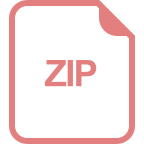
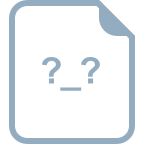
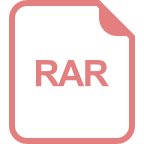
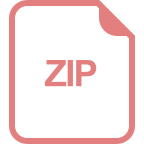
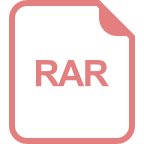
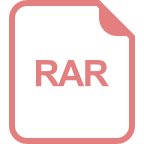
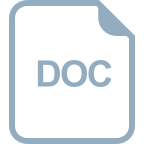
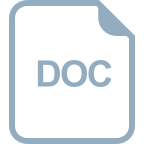
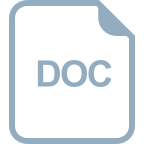
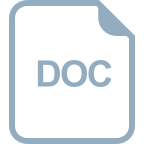
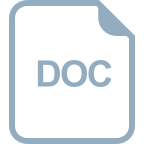
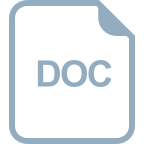
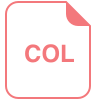
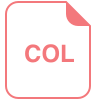
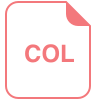
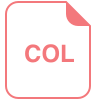
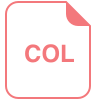