用python代码生成一个图书管理系统
时间: 2023-10-03 09:05:51 浏览: 64
好的,我可以给您提供一个基本的图书管理系统的Python代码,但是需要注意的是,这里提供的是一个简单的示例,实际应用中需要根据具体需求进行修改和完善。
```python
class Book:
def __init__(self, title, author, ISBN):
self.title = title
self.author = author
self.ISBN = ISBN
self.borrowed = False
class Library:
books = []
def add_book(self, book):
self.books.append(book)
def search_book(self, title):
for book in self.books:
if book.title == title:
return book
return None
def borrow_book(self, title):
book = self.search_book(title)
if book is not None and not book.borrowed:
book.borrowed = True
print(f"{title} has been borrowed.")
return True
else:
print(f"{title} is not available for borrowing.")
return False
def return_book(self, title):
book = self.search_book(title)
if book is not None and book.borrowed:
book.borrowed = False
print(f"{title} has been returned.")
else:
print(f"{title} is not a valid book to return.")
if __name__ == '__main__':
library = Library()
book1 = Book("The Hunger Games", "Suzanne Collins", "9780439023481")
book2 = Book("To Kill a Mockingbird", "Harper Lee", "9780446310789")
book3 = Book("1984", "George Orwell", "9780451524935")
library.add_book(book1)
library.add_book(book2)
library.add_book(book3)
print("Welcome to the library!")
while True:
print("What would you like to do?")
print("1. Search for a book")
print("2. Borrow a book")
print("3. Return a book")
print("4. Exit")
choice = input("Enter your choice: ")
if choice == "1":
title = input("Enter the title of the book: ")
book = library.search_book(title)
if book is not None:
print(f"{book.title} by {book.author} with ISBN {book.ISBN}")
else:
print(f"{title} is not available in the library.")
elif choice == "2":
title = input("Enter the title of the book: ")
library.borrow_book(title)
elif choice == "3":
title = input("Enter the title of the book: ")
library.return_book(title)
elif choice == "4":
print("Goodbye!")
break
else:
print("Invalid choice. Try again.")
```
这个简单的代码实现了一个图书馆的基本功能,包括添加书籍、搜索书籍、借出书籍和归还书籍等功能。
阅读全文
相关推荐
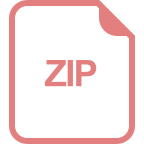
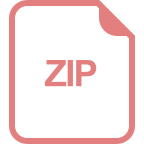


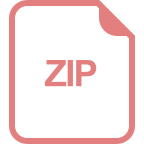









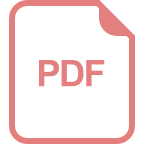
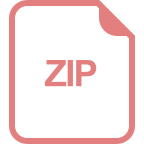
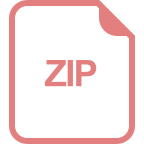
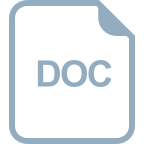