用 java.nio 包的类写一个服务端和一个客户端,客户端发送D盘文件 1.jpg给服务端,服务端接收文件,返回字符串“文件已接收”,并把文件保存为D盘的2.jpg
时间: 2023-09-15 21:06:00 浏览: 85
### 回答1:
以下是一个简单的示例代码,实现了用 java.nio 包的类写一个服务端和一个客户端,客户端发送D盘文件 1.jpg给服务端,服务端接收文件,返回字符串“文件已接收”,并把文件保存为D盘的2.jpg。
服务端代码:
```java
import java.io.FileOutputStream;
import java.nio.ByteBuffer;
import java.nio.channels.ServerSocketChannel;
import java.nio.channels.SocketChannel;
public class Server {
public static void main(String[] args) throws Exception {
ServerSocketChannel serverSocket = ServerSocketChannel.open();
serverSocket.socket().bind(new java.net.InetSocketAddress(8888));
while (true) {
SocketChannel client = serverSocket.accept();
ByteBuffer buffer = ByteBuffer.allocate(1024);
FileOutputStream fileOutputStream = new FileOutputStream("D:/2.jpg");
while (client.read(buffer) > 0) {
buffer.flip();
fileOutputStream.getChannel().write(buffer);
buffer.clear();
}
fileOutputStream.close();
client.close();
System.out.println("文件已接收");
}
}
}
```
客户端代码:
```java
import java.io.FileInputStream;
import java.nio.ByteBuffer;
import java.nio.channels.SocketChannel;
public class Client {
public static void main(String[] args) throws Exception {
SocketChannel client = SocketChannel.open();
client.connect(new java.net.InetSocketAddress("localhost", 8888));
FileInputStream fileInputStream = new FileInputStream("D:/1.jpg");
ByteBuffer buffer = ByteBuffer.allocate(1024);
while (fileInputStream.getChannel().read(buffer) > 0) {
buffer.flip();
client.write(buffer);
buffer.clear();
}
fileInputStream.close();
client.close();
System.out.println("发送成功");
}
}
```
注意:以上代码只是一个简单的示例,没有进行错误处理和完整性检查,实际应用中需要根据具体情况进行相应的处理。
### 回答2:
以下是用java.nio包编写的一个简单的服务端和客户端的代码,实现了客户端发送D盘文件1.jpg给服务端,服务端接收文件,返回字符串“文件已接收”,并把文件保存为D盘的2.jpg。
服务端代码(Server.java):
```java
import java.io.FileOutputStream;
import java.io.IOException;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.ServerSocketChannel;
import java.nio.channels.SocketChannel;
import java.nio.file.Path;
import java.nio.file.StandardOpenOption;
public class Server {
public static final int PORT = 8888;
public static final String SAVE_PATH = "D:\\2.jpg"; // 保存路径
public static void main(String[] args) throws IOException {
ServerSocketChannel serverChannel = ServerSocketChannel.open();
serverChannel.bind(new InetSocketAddress(PORT));
System.out.println("等待客户端连接...");
SocketChannel clientChannel = serverChannel.accept();
System.out.println("客户端已连接");
Path savePath = Path.of(SAVE_PATH);
try (FileOutputStream fos = new FileOutputStream(savePath.toFile())) {
ByteBuffer buffer = ByteBuffer.allocate(1024);
while (clientChannel.read(buffer) > 0) {
buffer.flip();
fos.getChannel().write(buffer);
buffer.clear();
}
} catch (IOException e) {
e.printStackTrace();
}
System.out.println("文件已接收并保存为2.jpg");
clientChannel.write(ByteBuffer.wrap("文件已接收".getBytes()));
clientChannel.close();
serverChannel.close();
}
}
```
客户端代码(Client.java):
```java
import java.io.FileInputStream;
import java.io.IOException;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.SocketChannel;
import java.nio.file.Path;
import java.nio.file.StandardOpenOption;
public class Client {
public static final String FILE_PATH = "D:\\1.jpg";
public static final int BUFFER_SIZE = 1024;
public static final String SERVER_IP = "127.0.0.1";
public static final int SERVER_PORT = 8888;
public static void main(String[] args) throws IOException {
SocketChannel socketChannel = SocketChannel.open();
socketChannel.connect(new InetSocketAddress(SERVER_IP, SERVER_PORT));
Path filePath = Path.of(FILE_PATH);
try (FileInputStream fis = new FileInputStream(filePath.toFile())) {
ByteBuffer buffer = ByteBuffer.allocate(BUFFER_SIZE);
int bytesRead;
while ((bytesRead = fis.getChannel().read(buffer)) > 0) {
buffer.flip();
socketChannel.write(buffer);
buffer.clear();
}
} catch (IOException e) {
e.printStackTrace();
}
System.out.println("文件发送完成");
ByteBuffer responseBuffer = ByteBuffer.allocate(BUFFER_SIZE);
socketChannel.read(responseBuffer);
System.out.println("服务端响应:" + new String(responseBuffer.array()));
socketChannel.close();
}
}
```
注意:以上代码仅实现了基本功能,还需要自行处理异常、文件不存在等情况。
### 回答3:
首先,我们可以使用Java的nio包中的SocketChannel类实现一个基于TCP协议的服务端和客户端之间的通信。下面是实现步骤:
1. 服务端代码:
```java
import java.io.FileOutputStream;
import java.io.IOException;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.ServerSocketChannel;
import java.nio.channels.SocketChannel;
public class Server {
public static void main(String[] args) {
try (ServerSocketChannel serverSocketChannel = ServerSocketChannel.open()) {
serverSocketChannel.bind(new InetSocketAddress(9999));
System.out.println("服务端已启动,等待客户端连接...");
SocketChannel socketChannel = serverSocketChannel.accept();
System.out.println("客户端已连接...");
ByteBuffer buffer = ByteBuffer.allocate(1024);
FileOutputStream fileOutputStream = new FileOutputStream("D:\\2.jpg");
int bytesRead;
while ((bytesRead = socketChannel.read(buffer)) != -1) {
buffer.flip();
while (buffer.hasRemaining()) {
fileOutputStream.write(buffer.get());
}
buffer.clear();
}
fileOutputStream.close();
socketChannel.close();
System.out.println("文件已接收,并保存为2.jpg");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
2. 客户端代码:
```java
import java.io.FileInputStream;
import java.io.IOException;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.SocketChannel;
public class Client {
public static void main(String[] args) {
try (SocketChannel socketChannel = SocketChannel.open()) {
socketChannel.connect(new InetSocketAddress("localhost", 9999));
ByteBuffer buffer = ByteBuffer.allocate(1024);
FileInputStream fileInputStream = new FileInputStream("D:\\1.jpg");
int bytesRead;
while ((bytesRead = fileInputStream.read(buffer.array())) != -1) {
buffer.flip();
socketChannel.write(buffer);
buffer.clear();
}
fileInputStream.close();
socketChannel.close();
System.out.println("文件发送完成!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
3. 使用命令行或者IDE编译并运行服务端和客户端代码。
4. 服务端接收到客户端发送的文件后,将其保存为D盘的2.jpg,并返回字符串"文件已接收"。
注意:在运行代码之前,请确保D盘上存在名为1.jpg的文件,并保证服务端和客户端代码在同一台机器上运行。
阅读全文
相关推荐
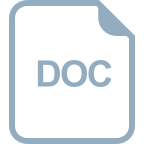
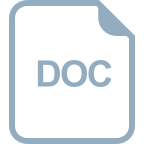
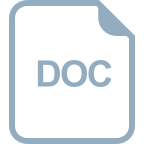


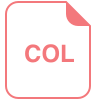
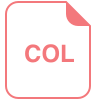
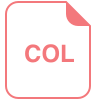
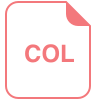
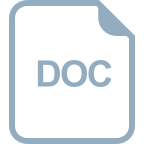
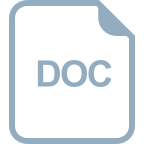
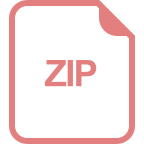
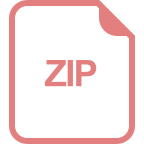