java nio tcp服务端接收 文字或者图片,接收到文字时打印,接收到图片时保存到D盘
时间: 2023-06-11 16:06:57 浏览: 141
以下是一个简单的 Java NIO TCP 服务端代码,可以接收客户端发送的文字或者图片,并进行相应处理:
```java
import java.io.FileOutputStream;
import java.io.IOException;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.ServerSocketChannel;
import java.nio.channels.SocketChannel;
import java.nio.charset.Charset;
public class NioTcpServer {
private static final int BUFFER_SIZE = 1024;
private static final Charset CHARSET = Charset.forName("UTF-8");
public static void main(String[] args) throws IOException {
// 创建 ServerSocketChannel 并绑定到指定端口
ServerSocketChannel serverSocketChannel = ServerSocketChannel.open();
serverSocketChannel.socket().bind(new InetSocketAddress(8080));
serverSocketChannel.configureBlocking(false);
while (true) {
// 接收客户端连接
SocketChannel socketChannel = serverSocketChannel.accept();
if (socketChannel != null) {
// 处理客户端请求
handleRequest(socketChannel);
}
}
}
private static void handleRequest(SocketChannel socketChannel) throws IOException {
ByteBuffer buffer = ByteBuffer.allocate(BUFFER_SIZE);
int bytesRead = socketChannel.read(buffer);
if (bytesRead == -1) {
// 客户端已关闭连接
socketChannel.close();
return;
}
buffer.flip();
byte[] bytes = new byte[buffer.remaining()];
buffer.get(bytes);
String message = new String(bytes, CHARSET);
if (message.startsWith("image:")) {
// 接收到图片,保存到 D 盘
String filename = message.substring("image:".length());
FileOutputStream fos = new FileOutputStream("D:\\" + filename);
while ((bytesRead = socketChannel.read(buffer)) != -1) {
buffer.flip();
fos.write(buffer.array(), 0, bytesRead);
buffer.clear();
}
fos.close();
} else {
// 接收到文字,打印
System.out.println(message);
}
}
}
```
客户端可以使用 telnet 来模拟发送请求:
- 发送文字:`telnet localhost 8080`,然后输入任意文字并回车即可。
- 发送图片:`telnet localhost 8080`,然后输入 `image:filename.jpg`,其中 `filename.jpg` 是图片文件名,然后将图片文件内容拷贝到 telnet 界面中发送即可。
阅读全文
相关推荐
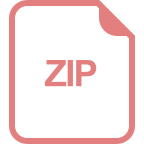
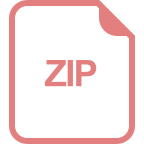
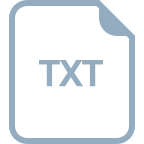
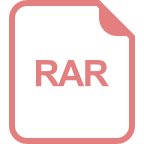
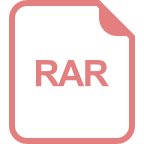
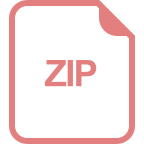
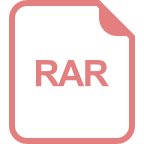
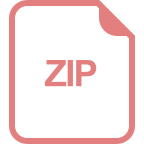
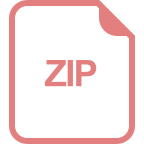
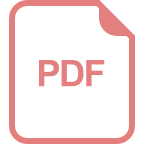
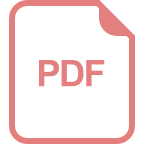
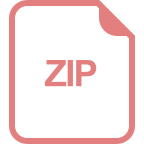