java socket用FileChannel把大文件分块无序发送到接收端代码
时间: 2024-04-14 18:30:10 浏览: 26
下面是一个示例代码,用于将大文件分块无序发送到接收端:
```java
import java.io.IOException;
import java.io.RandomAccessFile;
import java.net.ServerSocket;
import java.net.Socket;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
public class FileSender {
private static final int BLOCK_SIZE = 1024; // 每个文件块的大小
public static void sendFile(String filePath, String host, int port) {
try (ServerSocket serverSocket = new ServerSocket(port);
RandomAccessFile file = new RandomAccessFile(filePath, "r");
FileChannel channel = file.getChannel()) {
Socket socket = serverSocket.accept();
System.out.println("Accepted connection from: " + socket.getInetAddress());
ByteBuffer buffer = ByteBuffer.allocate(BLOCK_SIZE);
byte[] blockData = new byte[BLOCK_SIZE];
int bytesRead;
while ((bytesRead = channel.read(buffer)) != -1) {
buffer.flip();
buffer.get(blockData, 0, bytesRead);
sendBlock(socket, blockData, bytesRead);
buffer.clear();
}
socket.close();
System.out.println("File sent successfully.");
} catch (IOException e) {
e.printStackTrace();
}
}
private static void sendBlock(Socket socket, byte[] blockData, int length) throws IOException {
socket.getOutputStream().write(blockData, 0, length);
socket.getOutputStream().flush();
}
public static void main(String[] args) {
String filePath = "path/to/large_file.txt";
String host = "localhost";
int port = 12345;
sendFile(filePath, host, port);
}
}
```
在上述示例代码中,`FileSender`类使用`ServerSocket`来接受接收端的连接请求,并使用`RandomAccessFile`和`FileChannel`读取要发送的大文件。
在`sendFile()`方法中,首先创建`ServerSocket`并等待接收端的连接请求。一旦接收到连接,就会打印出连接的客户端信息。
然后,使用`ByteBuffer`和`FileChannel`按块读取文件数据,并将每个文件块通过`sendBlock()`方法发送到接收端。`sendBlock()`方法将文件块数据写入到Socket的输出流中。
最后,在示例代码的`main()`方法中,设置要发送的文件路径、接收端的主机和端口,并调用`sendFile()`方法开始发送文件。
请注意,上述示例代码仅演示了如何使用`FileChannel`将大文件分块无序发送到接收端。实际应用中,还需要考虑错误处理、超时设置等其他方面的逻辑。
相关推荐
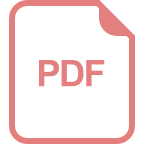
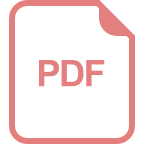
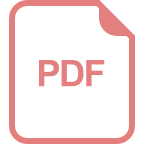
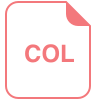
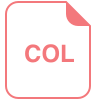
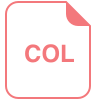
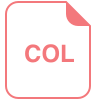
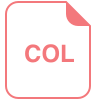









