FileChannel用MappedByteBuffer把一个大文件分块写入一个空文件中
时间: 2024-04-13 19:29:32 浏览: 205
要将一个大文件分块写入一个空文件中,您可以使用FileChannel和MappedByteBuffer来实现。以下是一个示例代码片段,演示了如何将大文件分块写入空文件中:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.nio.MappedByteBuffer;
import java.nio.channels.FileChannel;
public class FileChunkWriteExample {
public static void main(String[] args) {
String sourceFilePath = "path/to/source/file";
String destinationFilePath = "path/to/destination/file";
int bufferSize = 1024 * 1024; // 每个缓冲区的大小(1MB)
try (FileChannel sourceChannel = new FileInputStream(sourceFilePath).getChannel();
FileChannel destinationChannel = new FileOutputStream(destinationFilePath).getChannel()) {
long fileSize = sourceChannel.size();
long position = 0;
long remaining = fileSize;
while (remaining > 0) {
long chunkSize = Math.min(bufferSize, remaining);
MappedByteBuffer buffer = sourceChannel.map(FileChannel.MapMode.READ_ONLY, position, chunkSize);
destinationChannel.write(buffer);
position += chunkSize;
remaining -= chunkSize;
}
System.out.println("文件分块写入成功!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
请确保将示例代码中的"sourceFilePath"和"destinationFilePath"替换为实际的文件路径。在这个示例中,我们使用了一个1MB的缓冲区来分块读取源文件,并将每个缓冲区的数据写入目标文件中。您可以根据需要调整缓冲区的大小。
请注意,此示例代码没有处理异常情况,您可能需要进行适当的异常处理和错误检查。另外,如果目标文件已经存在,这段代码会覆盖目标文件的内容。如果您想追加数据而不是覆盖,请使用FileOutputStream的构造函数中传递第二个参数为`true`来打开文件输出流。
阅读全文
相关推荐
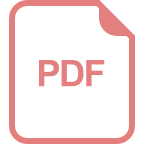
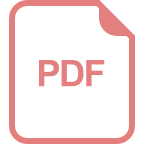


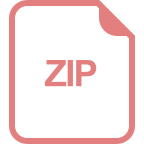
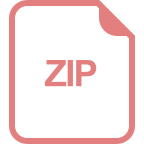
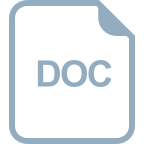
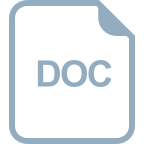
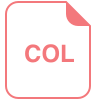
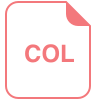
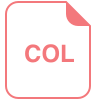
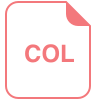
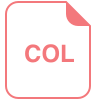
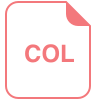
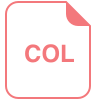
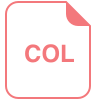


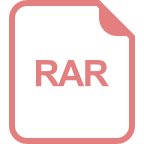