Java NIO中Channel的FileChannel实现文件IO与文件锁定
发布时间: 2024-02-12 06:37:06 阅读量: 17 订阅数: 11 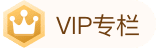
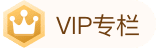
# 1. 介绍Java NIO中的Channel和FileChannel
## 1.1 了解Java NIO的概念
Java NIO(New I/O)是从Java 1.4版本开始引入的新一代I/O操作方式。它提供了对通道(Channel)和缓冲区(Buffer)的高效使用,以及非阻塞式IO特性。通道和缓冲区是NIO的核心概念,通过它们可以实现高效的数据传输和处理。
## 1.2 深入了解FileChannel的作用和特点
FileChannel是NIO中用于文件IO操作的通道,它可以实现文件的读取、写入和文件锁定等功能。FileChannel提供了一系列的方法来操作文件,相比传统的IO流,它具有更高的性能和更丰富的功能。
## 1.3 与传统IO的对比
传统的IO方式是基于流(InputStream和OutputStream)和阻塞式IO模型的。Java NIO中的FileChannel则采用了基于通道(Channel)和缓冲区(Buffer)的非阻塞式IO模型,相比传统IO,Java NIO具有更好的性能和可扩展性。
# 2. FileChannel实现文件读写操作
FileChannel是Java NIO中负责文件IO操作的核心类之一。它提供了一种高效的方式来进行文件的读写操作,并且相比传统的IO流,在处理大文件和并发访问时具有更好的性能优势。
### 2.1 使用FileChannel进行文件读操作
```java
import java.io.RandomAccessFile;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
public class FileReadExample {
public static void main(String[] args) {
try {
RandomAccessFile file = new RandomAccessFile("example.txt", "r");
FileChannel channel = file.getChannel();
// 创建缓冲区
ByteBuffer buffer = ByteBuffer.allocate(1024);
// 从FileChannel读取数据到缓冲区
int bytesRead = channel.read(buffer);
while (bytesRead != -1) {
// 切换缓冲区为读模式
buffer.flip();
// 读取缓冲区中的数据
while (buffer.hasRemaining()) {
System.out.print((char) buffer.get());
}
// 清空缓冲区,准备下一次读取
buffer.clear();
bytesRead = channel.read(buffer);
}
// 关闭FileChannel和RandomAccessFile
channel.close();
file.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
该示例代码实现了使用FileChannel进行文件读取的操作。首先,我们使用RandomAccessFile类创建一个对应于文件的RandomAccessFile对象,并获取其对应的FileChannel。然后,我们创建一个ByteBuffer作为缓冲区,大小为1024字节。通过调用FileChannel的read()方法读取数据到缓冲区中。读取完毕后,我们使用flip()方法切换缓冲区为读模式,并使用hasRemaining()和get()方法读取缓冲区中的数据并输出。最后,我们清空缓冲区并继续读取,直到读取到文件末尾。
### 2.2 使用FileChannel进行文件写操作
```java
import java.io.RandomAccessFile;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
public class FileWriteExample {
public static void main(String[] args) {
try {
RandomAccessFile file = new RandomAccessFile("example.txt", "rw");
FileChannel channel = file.getChannel();
// 创建缓冲区
ByteBuffer buffer = ByteBuffer.allocate(1024);
// 向缓冲区中写入数据
String data = "Hello, World!";
buffer.put(data.getBytes());
// 切换缓冲区为读模式
buffer.flip();
// 从缓冲区写入数据到FileChannel
channel.write(buffer);
// 关闭FileChannel和RandomAccessFile
channel.close();
file.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
该示例代码实现了使用FileChannel进行文件写入的操作。首先,我们使用RandomAccessFile类创建一个对应于文件的RandomAccessFile对象,并获取其对应的FileChannel。然后,我们创建一个ByteBuffer作为缓冲区,大小为1024字节。通过调用put()方法将数据写入缓冲区中。写入完毕后,我们使用flip()方法切换缓冲区为读模式,并调用write()方法将缓冲区中的数据写入到FileChannel中。最后,我们关闭FileChannel和RandomAccessFile。
### 2.3 文件复制和传输
```java
import java.io.RandomAccessFile;
import java.nio.channels.FileChannel;
public class FileCopyExample {
public static void main(String[] args) {
try {
RandomAccessFile sourceFile = new RandomAccessFile("source.txt", "r");
RandomAccessFile targetFile = new RandomAccessFile("target.txt", "rw");
FileChannel sourceChannel = sourceFile.getChannel();
FileChannel targetChannel = targetFile.getChannel();
// 使用transferTo()方法进行文件传输
targetChannel.transferFrom(sourceChannel, 0, sourceChannel.size());
// 关闭FileChannel和RandomAccessFile
sourceChannel.close();
```
0
0
相关推荐
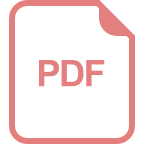
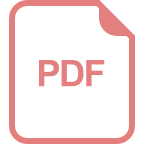
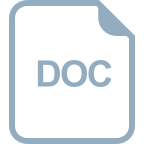
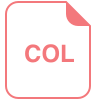
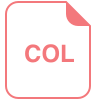
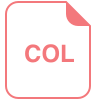
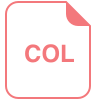

