利用R语言编写k折卷积神经网络图像回归算法
时间: 2024-01-25 20:43:09 浏览: 24
首先,我们需要导入必要的库和数据集。在这里,我们将使用“airquality”数据集来进行图像回归。
```R
library(keras)
library(tensorflow)
library(dplyr)
data("airquality")
```
接下来,我们需要对数据集进行预处理。由于卷积神经网络需要的输入是图像,因此我们需要将数据集转换为图像。我们可以使用ggplot2库将数据可视化为图像。
```R
library(ggplot2)
airquality %>%
ggplot(aes(x=Month, y=Day, fill=Ozone)) +
geom_tile() +
scale_fill_gradient(low="white", high="blue") +
theme_void() -> img
img <- as.raster(print(img, vp=viewport(width=4, height=4, units="in", clip="on")))
img <- image_to_matrix(img)[,,1]
```
在这里,我们将“airquality”数据集中的“Month”和“Day”作为图像的横纵坐标,将“Ozone”作为图像的颜色填充。然后,我们使用ggplot2库的“geom_tile”函数将数据可视化为热力图。接下来,我们使用“scale_fill_gradient”函数将颜色映射到白色到蓝色的颜色范围内。最后,我们使用“as.raster”函数将图像转换为光栅图像,并使用“image_to_matrix”函数将其转换为图像矩阵。在这里,我们只使用了R中的一个数据点来生成图像矩阵,但是在实际应用中,我们需要对整个数据集进行相同的操作。
接下来,我们将使用“image_data_generator”函数来生成训练数据和测试数据。在这里,我们将使用5个折叠来进行k折交叉验证。
```R
n_fold <- 5
batch_size <- 16
train_datagen <- image_data_generator(
rescale = 1/255,
validation_split = 1/n_fold
)
train_generator <- flow_images_from_data(
img_array = img,
generator = train_datagen,
batch_size = batch_size,
shuffle = TRUE,
subset = "training"
)
validation_generator <- flow_images_from_data(
img_array = img,
generator = train_datagen,
batch_size = batch_size,
shuffle = TRUE,
subset = "validation"
)
```
在这里,我们使用“rescale”参数将图像像素值的范围从0到255缩放到0到1之间。然后,我们使用“validation_split”参数将数据集分成训练集和验证集。接下来,我们使用“flow_images_from_data”函数将数据集转换为可以用于训练的数据生成器。
接下来,我们将定义一个卷积神经网络模型。在这里,我们将使用一个包含3个卷积层和3个池化层的模型。
```R
model <- keras_model_sequential() %>%
layer_conv_2d(filters = 32, kernel_size = c(3, 3), activation = "relu", input_shape = dim(img)) %>%
layer_max_pooling_2d(pool_size = c(2, 2)) %>%
layer_conv_2d(filters = 64, kernel_size = c(3, 3), activation = "relu") %>%
layer_max_pooling_2d(pool_size = c(2, 2)) %>%
layer_conv_2d(filters = 128, kernel_size = c(3, 3), activation = "relu") %>%
layer_max_pooling_2d(pool_size = c(2, 2)) %>%
layer_flatten() %>%
layer_dense(units = 128, activation = "relu") %>%
layer_dropout(rate = 0.5) %>%
layer_dense(units = 1, activation = "linear")
summary(model)
```
在这里,我们使用“keras_model_sequential”函数创建了一个序列模型。然后,我们使用“layer_conv_2d”函数添加3个卷积层和“layer_max_pooling_2d”函数添加3个池化层。接下来,我们使用“layer_flatten”函数将卷积层的输出展平,并使用“layer_dense”函数添加2个全连接层。最后,我们使用“layer_dropout”函数添加一个dropout层,并使用“layer_dense”函数添加一个输出层。
在定义好模型后,我们需要编译模型并训练模型。
```R
model %>% compile(
optimizer = optimizer_rmsprop(),
loss = "mse",
metrics = c("mae")
)
history <- model %>% fit_generator(
generator = train_generator,
validation_data = validation_generator,
steps_per_epoch = 50,
epochs = 10
)
```
在这里,我们使用“optimizer_rmsprop”函数作为优化器,并使用“mse”作为损失函数。我们还使用“mae”作为度量指标。然后,我们使用“fit_generator”函数训练模型。在这里,我们使用“train_generator”作为训练数据生成器,使用“validation_generator”作为验证数据生成器,使用“steps_per_epoch”参数指定每个epoch中使用的训练步数,使用“epochs”参数指定训练的epoch数。
最后,我们可以使用“evaluate_generator”函数评估模型的性能。
```R
model %>% evaluate_generator(
generator = validation_generator,
steps = 50
)
```
在这里,我们使用“validation_generator”作为验证数据生成器,并使用“steps”参数指定评估模型时使用的步数。
相关推荐
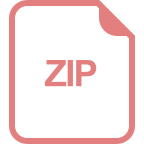
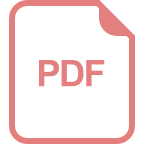
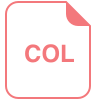
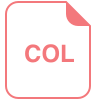
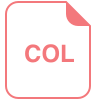
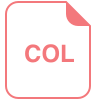
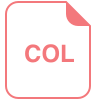









