proto有三个message 保存数据到文件时应该怎么保存 请以Android studio java语言举例
时间: 2024-11-05 11:24:36 浏览: 32
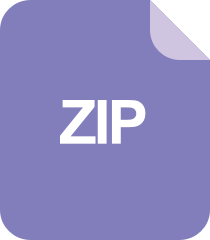
MacOS系统proto文件转Java
在Android Studio中使用Java语言处理Protocol Buffers (protobuf)保存数据到文件,你可以按照以下步骤操作:
1. 首先,确保你已经添加了protobuf库到项目。如果你还没做,可以在build.gradle(Module: app)中添加依赖,例如:
```groovy
dependencies {
implementation 'com.google.protobuf:protobuf-java:3.17.0'
}
```
2. 定义你的Proto消息结构(`Message.proto`):
```proto
syntax = "proto3";
message DataMessage {
string key = 1;
bytes value = 2;
}
message FileData {
repeated DataMessage dataItems = 1;
}
```
这里假设有一个名为`FileData`的消息类型,包含了`DataMessage`类型的列表。
3. 使用`ProtobufIO`和`ByteString`来序列化和反序列化消息:
```java
import com.google.protobuf.ByteString;
import com.google.protobuf.InvalidProtocolBufferException;
import com.google.protobuf.TextFormat;
public void saveToFile(FileData fileData, String filePath) throws IOException {
// 将FileData对象序列化成字节串
ByteString byteString = TextFormat.printToString(fileData);
// 写入文件
FileOutputStream fos = new FileOutputStream(filePath);
fos.write(byteString.toByteArray());
fos.close();
}
public FileData loadFromFile(String filePath) throws IOException, InvalidProtocolBufferException {
// 从文件读取字节串
FileInputStream fis = new FileInputStream(filePath);
byte[] bytes = fis.readAllBytes();
// 反序列化字节串回FileData对象
return FileData.parseFrom(bytes);
}
```
4. 调用上述方法保存和加载数据:
```java
try {
FileData data = FileData.newBuilder()
.addDataItems(DataMessage.newBuilder().setKey("key").setValue(ByteString.copyFromUtf8("value"))))
.build();
saveToFile(data, "data.pb");
FileData loadedData = loadFromFile("data.pb");
System.out.println(loadedData.getDataItemsCount()); // 输出:1 或者其他实际的数量
} catch (IOException | InvalidProtocolBufferException e) {
e.printStackTrace();
}
```
阅读全文
相关推荐
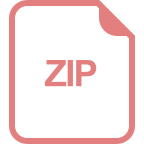















