判断单链表L(带头结点)是否为递增的 c语言实现
时间: 2024-09-27 16:09:00 浏览: 4
判断单链表L(带头结点)是否为递增的,可以遍历链表,比较当前节点的值与前一个节点的值。如果对于链表中的每个相邻节点,当前节点的值都大于前一个节点的值,那么链表就是递增的。以下是一个简单的C语言实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct ListNode {
int val;
struct ListNode* next;
} ListNode;
// 检查链表是否递增
int isMonotonicIncreasing(ListNode* head) {
if (head == NULL || head->next == NULL) { // 如果链表为空或者只有一个元素
return 1; // 都视为递增
}
ListNode* current = head->next;
while (current != NULL) {
if (current->val <= head->val) { // 当前节点值不大于前一节点,不是递增
return 0;
}
head = current; // 更新头指针到下一个节点
current = current->next;
}
return 1; // 遍历结束,链表递增
}
// 创建并初始化链表示例
ListNode* createList(int* arr, int size) {
ListNode* head = (ListNode*)malloc(sizeof(ListNode));
head->val = arr[0];
head->next = NULL;
ListNode* temp = head;
for (int i = 1; i < size; i++) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
newNode->val = arr[i];
newNode->next = temp->next;
temp->next = newNode;
temp = newNode;
}
return head;
}
int main() {
int arr[] = {1, 2, 4, 6};
int n = sizeof(arr) / sizeof(arr[0]);
ListNode* list = createList(arr, n);
if (isMonotonicIncreasing(list)) {
printf("链表是递增的\n");
} else {
printf("链表不是递增的\n");
}
freeListNode(list); // 自定义链表释放函数
return 0;
}
相关推荐
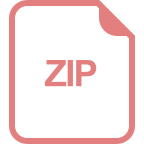
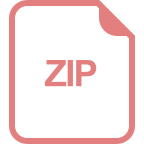
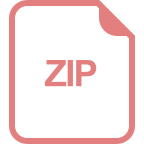



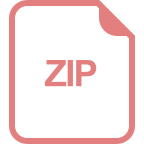


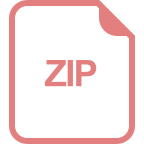
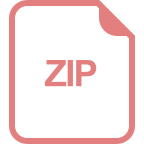
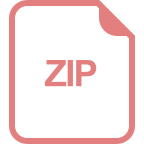
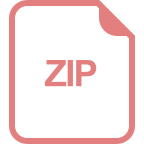
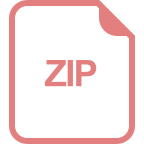
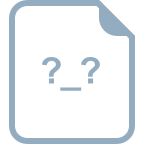