c语言求两递增有序的带头节点的单链表A,B分别表示一个集合,求这两个集合的交集并赋予A
时间: 2024-09-07 15:04:54 浏览: 66
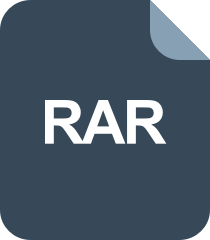
2_链表_求la和lb的交集_

在C语言中,求两个递增有序的单链表A和B的交集,并将结果合并到A链表上,可以采用遍历和比较的方式实现。这里是一个简化的步骤:
1. 定义链表结构(如果有头结点):
```c
typedef struct ListNode {
int data;
struct ListNode* next;
} ListNode;
```
2. 创建辅助函数来判断是否为交集元素:
```c
ListNode* findIntersection(ListNode* A, ListNode* B) {
ListNode* p1 = A;
ListNode* p2 = B;
while (p1 != NULL && p2 != NULL) {
if (p1->data == p2->data) {
return p1; // 找到了相同的元素
}
p1 = (p1->next) ? p1->next : A; // 如果p1到达了尾部,重新从A开始
p2 = (p2->next) ? p2->next : B; // 同理,如果p2到达尾部,重新从B开始
}
return NULL; // 没有找到交集元素
}
```
3. 更新A链表的交集部分:
```c
void mergeIntersection(ListNode* A, ListNode* intersection) {
ListNode* temp = intersection;
while (temp != NULL) {
// 遍历A链表,查找是否存在相同数据,然后插入
ListNode* current = A;
while (current != NULL && current->data < temp->data) {
current = current->next;
}
if (current != NULL && current->data == temp->data) {
temp = temp->next; // 跳过已存在的元素
continue;
}
// 插入交集元素到A链表
ListNode* newNode = malloc(sizeof(ListNode));
newNode->data = temp->data;
newNode->next = current->next;
current->next = newNode;
}
}
```
4. 总体流程:
```c
ListNode* result = findIntersection(A, B);
if (result != NULL) {
mergeIntersection(A, result);
}
```
阅读全文
相关推荐
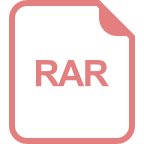
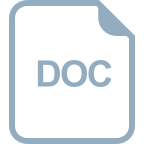

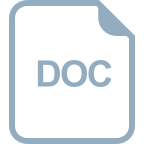
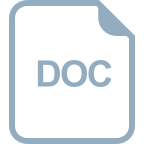












