使用C语言,假设以两个元素依值递增有序排列的线性表 分别表示两个集合(即 同一表中的元素值各不相同),现要求另辟空间构成一个线性表 ,其元素为 中元 素的交集,且表 中的元素也依值递增有序排列。试对单链表编写求 的算法
时间: 2023-05-30 07:05:20 浏览: 129
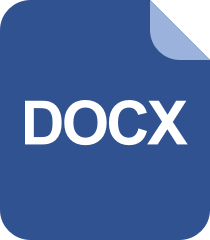
数据结构 两个有序线性表的归并算法 西南交通大学
算法思路:
1. 遍历两个有序链表,比较当前节点的值大小,将值小的节点指针向后移动。
2. 如果两个节点的值相等,将该节点的值插入到新的链表中,并将两个链表的节点指针都向后移动。
3. 直到其中一个链表遍历结束,则交集已经全部找到。
4. 返回新的链表头节点。
C语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* next;
} Node, *LinkedList;
LinkedList createLinkedList(int arr[], int n) {
LinkedList head = (LinkedList)malloc(sizeof(Node));
head->next = NULL;
Node* p = head;
for (int i = 0; i < n; i++) {
Node* node = (Node*)malloc(sizeof(Node));
node->data = arr[i];
node->next = NULL;
p->next = node;
p = node;
}
return head;
}
LinkedList findIntersection(LinkedList list1, LinkedList list2) {
LinkedList head = (LinkedList)malloc(sizeof(Node));
head->next = NULL;
Node* p = head;
Node* p1 = list1->next;
Node* p2 = list2->next;
while (p1 != NULL && p2 != NULL) {
if (p1->data < p2->data) {
p1 = p1->next;
} else if (p1->data > p2->data) {
p2 = p2->next;
} else {
Node* node = (Node*)malloc(sizeof(Node));
node->data = p1->data;
node->next = NULL;
p->next = node;
p = node;
p1 = p1->next;
p2 = p2->next;
}
}
return head;
}
void printLinkedList(LinkedList head) {
Node* p = head->next;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
int main() {
int arr1[] = {1, 3, 5, 7};
int arr2[] = {2, 3, 4, 7, 8};
LinkedList list1 = createLinkedList(arr1, 4);
LinkedList list2 = createLinkedList(arr2, 5);
LinkedList list = findIntersection(list1, list2);
printLinkedList(list);
return 0;
}
```
阅读全文
相关推荐
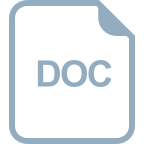
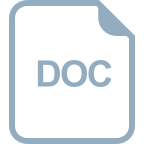





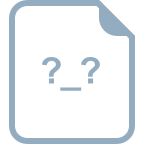
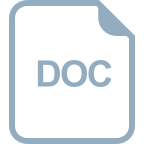
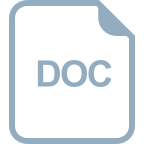
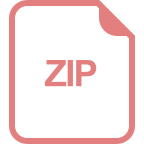
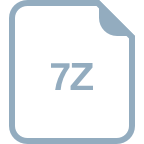
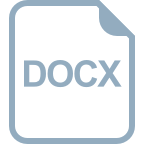
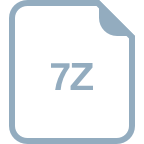