已知两个链表A和B分别表示两个集合,其中元素递增排列,设计一个算法,用于求A与B的交集,并放在A中。c语言完整代码实现。
时间: 2024-03-19 22:39:20 浏览: 103
以下是用C语言实现求两个递增链表的交集并放在A中的算法:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建链表
Node* createList(int arr[], int size) {
Node* head = NULL;
Node* tail = NULL;
for (int i = 0; i < size; i++) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = arr[i];
newNode->next = NULL;
if (head == NULL) {
head = newNode;
tail = newNode;
} else {
tail->next = newNode;
tail = newNode;
}
}
return head;
}
// 求两个递增链表的交集并放在A中
void intersect(Node** A, Node* B) {
Node* p1 = *A;
Node* p2 = B;
Node* prev = NULL;
while (p1 != NULL && p2 != NULL) {
if (p1->data < p2->data) {
prev = p1;
p1 = p1->next;
} else if (p1->data > p2->data) {
p2 = p2->next;
} else {
if (prev == NULL) {
*A = p1->next;
} else {
prev->next = p1->next;
}
Node* temp = p1;
p1 = p1->next;
free(temp);
}
}
}
// 打印链表
void printList(Node* head) {
Node* p = head;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
int main() {
int arr1[] = {1, 2, 3, 4, 5};
int arr2[] = {3, 4, 5, 6, 7};
Node* A = createList(arr1, sizeof(arr1) / sizeof(arr1[0]));
Node* B = createList(arr2, sizeof(arr2) / sizeof(arr2[0]));
printf("链表A:");
printList(A);
printf("链表B:");
printList(B);
intersect(&A, B);
printf("交集放在链表A中:");
printList(A);
return 0;
}
```
阅读全文
相关推荐
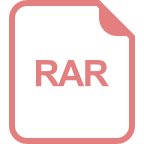
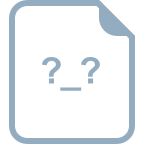















