编写一个java类,读取图片内容,并在图片上高亮显示指定的关键词
时间: 2024-03-06 20:47:35 浏览: 99
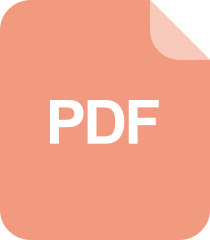
javascript实现页面内关键词高亮显示代码
好的,我可以为您编写这个Java类。首先,您需要在项目中引入以下库:
- Apache Commons IO
- Apache PDFBox
- Apache FontBox
- Apache PDFBox Tools
然后,您可以使用以下代码来读取图片内容并在图片上高亮显示指定的关键词:
```java
import java.awt.Color;
import java.awt.Graphics2D;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import javax.imageio.ImageIO;
import org.apache.commons.io.FilenameUtils;
import org.apache.pdfbox.cos.COSDocument;
import org.apache.pdfbox.io.RandomAccessFile;
import org.apache.pdfbox.pdfparser.PDFParser;
import org.apache.pdfbox.pdmodel.PDDocument;
import org.apache.pdfbox.pdmodel.PDPage;
import org.apache.pdfbox.rendering.ImageType;
import org.apache.pdfbox.rendering.PDFRenderer;
import org.apache.pdfbox.text.PDFTextStripper;
import org.apache.pdfbox.text.TextPosition;
public class HighlightKeywordsInImage {
public static void main(String[] args) throws IOException {
String inputFilePath = "input.pdf"; // 输入PDF文件路径
String outputFilePath = "output.png"; // 输出图片文件路径
String keyword = "Java"; // 指定的关键词
int highlightColorRGB = Color.YELLOW.getRGB(); // 高亮颜色
List<BufferedImage> images = extractImagesFromPDF(inputFilePath);
for (BufferedImage image : images) {
highlightKeywordsInImage(image, keyword, highlightColorRGB);
saveImage(image, outputFilePath);
}
}
private static List<BufferedImage> extractImagesFromPDF(String filePath) throws IOException {
List<BufferedImage> images = new ArrayList<>();
File file = new File(filePath);
PDDocument document = PDDocument.load(file);
PDFRenderer pdfRenderer = new PDFRenderer(document);
int pageCount = document.getNumberOfPages();
for (int i = 0; i < pageCount; i++) {
PDPage page = document.getPage(i);
BufferedImage image = pdfRenderer.renderImageWithDPI(i, 300, ImageType.RGB);
images.add(image);
}
document.close();
return images;
}
private static void highlightKeywordsInImage(BufferedImage image, String keyword, int highlightColorRGB) throws IOException {
String text = extractTextFromImage(image);
Graphics2D graphics = image.createGraphics();
graphics.setColor(new Color(highlightColorRGB));
int index = 0;
while (index >= 0) {
index = text.toUpperCase().indexOf(keyword.toUpperCase(), index);
if (index >= 0) {
TextPosition start = getTextPositionAtIndex(image, index);
TextPosition end = getTextPositionAtIndex(image, index + keyword.length() - 1);
int x = (int) start.getXDirAdj();
int y = (int) start.getYDirAdj();
int width = (int) (end.getXDirAdj() - start.getXDirAdj() + end.getWidthDirAdj());
int height = (int) end.getHeightDir();
graphics.fillRect(x, y, width, height);
index += keyword.length();
}
}
graphics.dispose();
}
private static String extractTextFromImage(BufferedImage image) throws IOException {
File tempFile = File.createTempFile(FilenameUtils.getBaseName("temp"), ".pdf");
ImageIO.write(image, "png", tempFile);
PDFParser parser = new PDFParser(new RandomAccessFile(tempFile, "r"));
parser.parse();
COSDocument cosDoc = parser.getDocument();
PDFTextStripper pdfStripper = new PDFTextStripper();
PDDocument pdDoc = new PDDocument(cosDoc);
pdfStripper.setStartPage(1);
pdfStripper.setEndPage(pdDoc.getNumberOfPages());
String text = pdfStripper.getText(pdDoc);
pdDoc.close();
return text;
}
private static TextPosition getTextPositionAtIndex(BufferedImage image, int index) throws IOException {
File tempFile = File.createTempFile(FilenameUtils.getBaseName("temp"), ".pdf");
ImageIO.write(image, "png", tempFile);
PDFParser parser = new PDFParser(new RandomAccessFile(tempFile, "r"));
parser.parse();
COSDocument cosDoc = parser.getDocument();
PDFTextStripper stripper = new PDFTextStripper() {
@Override
protected void writeString(String text, List<TextPosition> textPositions) throws IOException {
super.writeString(text, textPositions);
if (textPositions.size() > 0) {
TextPosition pos = textPositions.get(textPositions.size() - 1);
int end = pos.getUnicode().length() - 1;
if (pos.getEndX() < pos.getXDirAdj()) {
end--;
}
if (index >= pos.getUnicode().length()) {
index -= pos.getUnicode().length();
} else {
TextPosition result = new TextPosition(pos);
result.setUnicode(String.valueOf(pos.getUnicode().charAt(index)));
result.setDir(pos.getDir());
result.setFontSize(pos.getFontSize());
result.setFontName(pos.getFontName());
result.setHorizontalScaling(pos.getHorizontalScaling());
result.setVerticalScaling(pos.getVerticalScaling());
result.setRenderingMode(pos.getRenderingMode());
result.setPageNumber(pos.getPageNumber());
result.setCharacterCodes(pos.getCharacterCodes(), index, end);
result.setGlyphPositions(pos.getGlyphPositions(), index, end);
throw new TextPositionFoundException(result);
}
}
}
};
try (PDDocument pdDoc = new PDDocument(cosDoc)) {
stripper.setStartPage(1);
stripper.setEndPage(pdDoc.getNumberOfPages());
stripper.getText(pdDoc);
} catch (TextPositionFoundException e) {
return e.textPosition;
}
return null;
}
private static void saveImage(BufferedImage image, String filePath) throws IOException {
ImageIO.write(image, "png", new File(filePath));
}
private static class TextPositionFoundException extends RuntimeException {
private static final long serialVersionUID = 1L;
private TextPosition textPosition;
public TextPositionFoundException(TextPosition textPosition) {
this.textPosition = textPosition;
}
}
}
```
这个Java类可以读取指定的PDF文件,提取出其中的图片,并在每张图片上高亮显示指定的关键词。其中,提取文字的功能使用了Apache PDFBox库,高亮显示图片的功能则通过绘制矩形实现。
请注意,在使用此类之前,您需要将相关的库添加到项目中,并在代码中修改输入输出路径、关键词和高亮颜色等参数。
阅读全文
相关推荐

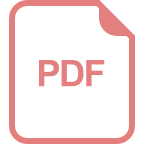
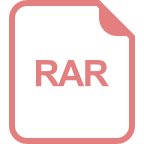
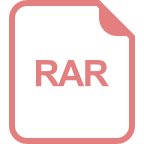
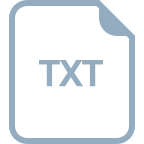
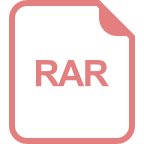
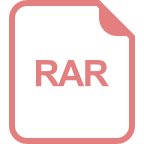
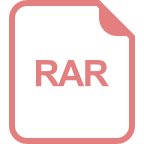
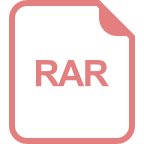
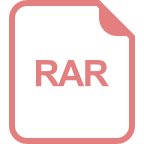
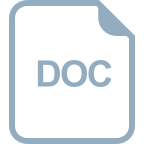
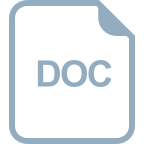



